c语言串口队列收发代码
时间: 2024-09-19 21:17:53 浏览: 77
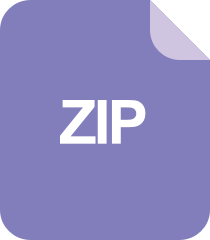
51单片机串口接收使用队列C语言实现

在C语言中,处理串口通信的队列收发通常涉及到硬件访问和操作系统调度。这里给出一个基本的示例,使用FIFO(First-In-First-Out,先进先出)队列作为数据传输通道:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <termios.h>
#define SERIAL_PORT "/dev/ttyUSB0"
#define BUFFER_SIZE 100
typedef struct {
char data[BUFFER_SIZE];
int head;
int tail;
} SerialQueue;
void enqueue(SerialQueue *queue, const char *msg) {
if (queue->tail == queue->head) {
printf("Queue is full, cannot enqueue.\n");
return;
}
strncpy(queue->data + queue->tail, msg, BUFFER_SIZE - queue->tail);
queue->tail = (queue->tail + 1) % BUFFER_SIZE;
}
char dequeue(SerialQueue *queue) {
if (queue->head == queue->tail) {
printf("Queue is empty, cannot dequeue.\n");
return '\0';
}
char ret = queue->data[queue->head];
queue->head = (queue->head + 1) % BUFFER_SIZE;
return ret;
}
int main() {
SerialQueue serialQueue;
serialQueue.head = serialQueue.tail = 0;
// Open the serial port
int fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("Failed to open serial port");
return 1;
}
// Configure the serial settings
struct termios options;
tcgetattr(fd, &options);
options.c_cflag &= ~CSIZE; // Set character size to 8 bits
options.c_cflag |= CS8; // Enable 8-bit mode
options.c_iflag &= ~(IXON | IXOFF); // Disable hardware flow control
options.c_oflag &= ~OPOST; // Disable sending of characters when CTS/RTS signals are active
options.c_lflag &= ~(ICANON | ECHO); // Disable line buffering and echo
tcflush(fd, TCIFLUSH); // Flush input buffer
tcsetattr(fd, TCSANOW, &options);
// Main loop for sending and receiving
while (true) {
char message[256];
fgets(message, sizeof(message), stdin);
enqueue(&serialQueue, message);
if (!dequeue(&serialQueue)) continue; // If no data to send
write(fd, serialQueue.data, strlen(serialQueue.data) + 1); // Send data over the serial port
}
close(fd);
return 0;
}
```
这个代码首先打开串口设备,设置通信参数,然后在一个无限循环中接收用户输入,并把数据入队;同时检查队列是否有待发送的数据,如有则发送。
阅读全文
相关推荐
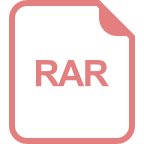
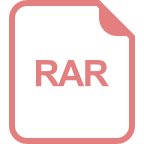

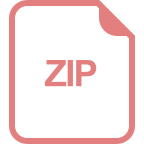
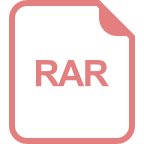
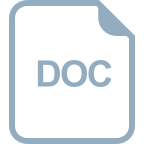
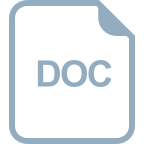
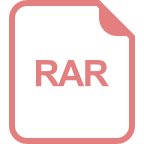
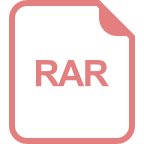
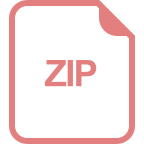
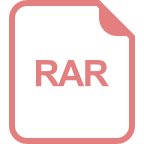
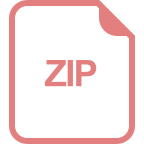
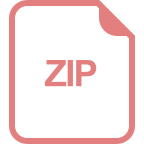
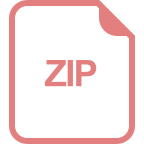
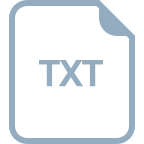
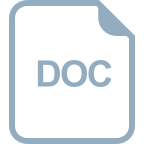
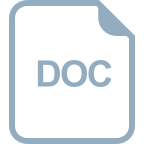

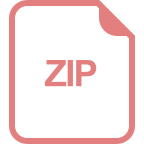