用C语言实现消息队列的通信
时间: 2024-02-16 17:04:55 浏览: 28
要使用C语言实现消息队列的通信,可以通过以下步骤进行:
1. 包含相关的头文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#include <errno.h>
```
2. 定义消息队列结构体:
```c
struct msgbuf {
long mtype; // 消息类型
char mtext[1024]; // 消息内容
};
```
3. 创建或获取消息队列的ID:
```c
key_t key = ftok(".", 'a'); // 获取键值
int msqid = msgget(key, 0666 | IPC_CREAT); // 创建或获取消息队列ID
if (msqid == -1) {
perror("msgget");
exit(1);
}
```
4. 向消息队列中发送消息:
```c
struct msgbuf msg;
msg.mtype = 1; // 消息类型为1
strcpy(msg.mtext, "Hello world!"); // 消息内容
if (msgsnd(msqid, &msg, strlen(msg.mtext) + 1, 0) == -1) {
perror("msgsnd");
exit(1);
}
```
5. 从消息队列中接收消息:
```c
struct msgbuf msg;
if (msgrcv(msqid, &msg, sizeof(msg.mtext), 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message: %s\n", msg.mtext);
```
6. 删除消息队列:
```c
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
```
以上就是使用C语言实现消息队列的通信的基本步骤。需要注意的是,在实际应用中还需要考虑消息的格式、长度、错误处理等问题。
相关推荐
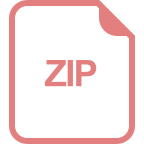
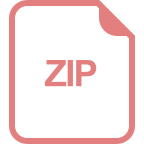
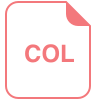
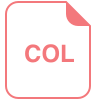
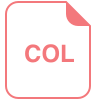
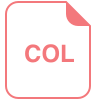
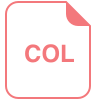









