使用C语言编写程序设计两个独立进程,一个是发送进程,一个是接收进程,通过消息队列进行进程通信,以“end”作为结束消息。
时间: 2023-06-13 09:06:58 浏览: 139
好的,以下是使用消息队列进行进程通信的 C 语言代码:
send.c:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
typedef struct {
long msg_type;
char msg_text[MSG_SIZE];
} Message;
int main() {
Message message;
int msgid;
// 创建消息队列
msgid = msgget((key_t)1234, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
printf("Enter message to send: ");
fgets(message.msg_text, MSG_SIZE, stdin);
// 发送消息
message.msg_type = 1;
while (strncmp(message.msg_text, "end", 3) != 0) {
if (msgsnd(msgid, (void *)&message, MSG_SIZE, 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
printf("Enter message to send: ");
fgets(message.msg_text, MSG_SIZE, stdin);
}
// 删除消息队列
if (msgctl(msgid, IPC_RMID, 0) == -1) {
perror("msgctl failed");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
```
recv.c:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
typedef struct {
long msg_type;
char msg_text[MSG_SIZE];
} Message;
int main() {
Message message;
int msgid;
// 获取消息队列
msgid = msgget((key_t)1234, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
while (1) {
// 接收消息
if (msgrcv(msgid, (void *)&message, MSG_SIZE, 0, 0) == -1) {
perror("msgrcv failed");
exit(EXIT_FAILURE);
}
printf("Received message: %s", message.msg_text);
// 判断是否结束
if (strncmp(message.msg_text, "end", 3) == 0) {
break;
}
}
exit(EXIT_SUCCESS);
}
```
以上代码中,send.c 文件创建了一个消息队列,并循环从标准输入中读取消息并发送到消息队列中,直到读取到了 "end" 消息为止。recv.c 文件获取了同样的消息队列,并循环从消息队列中接收消息并打印到标准输出中,直到接收到了 "end" 消息为止。
相关推荐
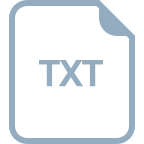
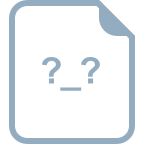














