C#实现多张图片合并到一个字节数组保存到sqlsever中的一个字段,在从数据库读取回来拆分保存成多张图片,提供完整示例代码
时间: 2024-10-09 16:13:15 浏览: 49
在C#中,你可以使用`System.Drawing.Image`和`System.Data.SqlClient`库来处理将多张图片合并到SQL Server的`VARBINARY(MAX)`类型的字段中,然后从数据库读取并拆分开。以下是步骤和一个简单的示例:
首先,你需要安装`System.Drawing.Common` NuGet包,它包含了处理图像的所需功能。
```csharp
using System;
using System.Data;
using System.Data.SqlClient;
using System.IO;
public class ImageHandler
{
public static void SaveImagesToSql(byte[] imagesData, SqlConnection connection)
{
// 创建一个SqlConnection实例
string connectionString = "your_connection_string_here";
using (SqlConnection dbConnection = new SqlConnection(connectionString))
{
if (!dbConnection.Open()) throw new Exception("Failed to open database connection.");
// 将所有图片数据合并到一个BLOB
byte[] combinedImageData = CombineImages(imagesData);
// 创建SqlCommand对象
SqlCommand command = new SqlCommand("INSERT INTO YourTable (ImageField) VALUES (@CombinedImage)", dbConnection);
command.Parameters.AddWithValue("@CombinedImage", SqlDbType.VarBinary).Value = combinedImageData;
// 执行插入操作
int rowsAffected = command.ExecuteNonQuery();
if (rowsAffected <= 0) throw new Exception("Failed to save the image(s) to the database.");
}
}
private static byte[] CombineImages(byte[][] imageArrays)
{
// 假设所有的图片数组都在一个列表里
var imagesList = new List<byte[]>(imageArrays);
// 将所有图片数据追加到一个大的字节数组
var combinedData = new byte[0];
foreach (var imageData in imagesList)
{
combinedData = Array.Concat(combinedData, imageData);
}
return combinedData;
}
public static byte[][] RetrieveImagesFromSql(SqlConnection connection)
{
// 从数据库获取BLOB数据
string sqlQuery = "SELECT ImageField FROM YourTable";
using (SqlCommand command = new SqlCommand(sqlQuery, connection))
{
byte[] combinedImageData = (byte[])command.ExecuteScalar();
// 拆分图片数据
return SplitCombinedImage(combinedImageData);
}
}
private static byte[][] SplitCombinedImage(byte[] combinedImageData)
{
// 这里只是一个基本的示例,实际应用中需要处理分割逻辑,例如每4字节可能是新的一帧或一个压缩块
// 这部分取决于你的图片数据结构
byte[][] result = new byte[combinedImageData.Length / 4][];
for (int i = 0; i < result.Length; i++)
{
result[i] = new byte[4];
Buffer.BlockCopy(combinedImageData, i * 4, result[i], 0, 4);
}
return result;
}
}
// 使用示例
string connectionString = "your_connection_string_here";
byte[][] imagesArray = ... // 你的图片数据数组
SqlConnection dbConnection = new SqlConnection(connectionString);
try
{
ImageHandler.SaveImagesToSql(imagesArray, dbConnection);
// 数据已成功存储
// 从数据库检索
byte[][] retrievedImages = ImageHandler.RetrieveImagesFromSql(dbConnection);
// 现在你可以对retrievedImages进行操作,如保存到文件或其他用途
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
```
请注意,这个示例假设每个图片的数据是以独立的字节数组形式传入,并且你有一个合适的方式来确定如何在数据库中正确地解析和恢复这些数据。实际应用中,你可能需要使用更复杂的方法,比如二进制流或特定的图像格式(如JPEG或PNG)。
阅读全文
相关推荐
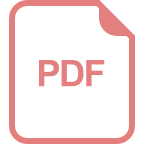
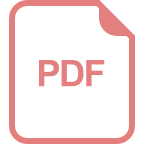
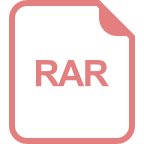

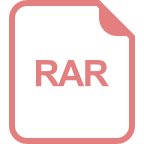
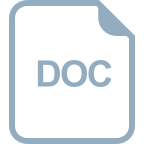
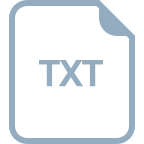
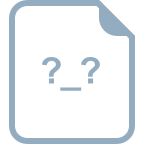
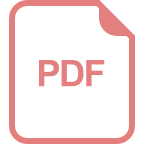
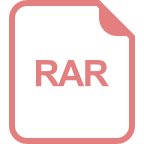
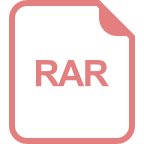
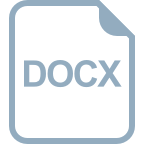
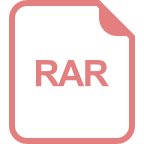
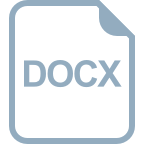
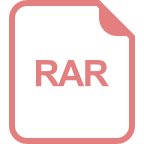
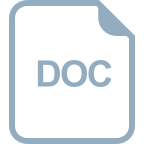
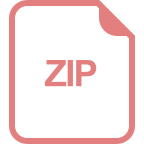
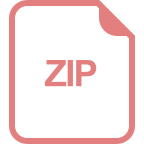
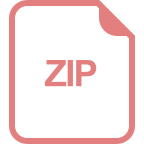