1、定义一个单向链表的模板类,分别实现增加、删除、查找和打印操作。要求在main函数中由链表模板类创建整型类模板并测试各个成员函数。
时间: 2024-10-19 20:10:31 浏览: 38
首先,我们创建一个基础的单向链表模板类`ListNode`,包含节点数据和指向下一个节点的指针。然后定义四个基本操作:插入节点、删除节点、查找节点以及打印链表。这里假设整数`int`作为链表元素。
```cpp
template <typename T>
class ListNode {
public:
T data; // 节点的数据
ListNode<T>* next; // 指向下一个节点的指针
// 构造函数
ListNode(T value) : data(value), next(nullptr) {}
// 插入节点
void insertNode(const T& value) {
if (next == nullptr) {
next = new ListNode<T>(value);
} else {
ListNode<T>* newNode = new ListNode<T>(value);
newNode->next = next;
next = newNode;
}
}
// 删除节点
bool deleteNode(T value) {
if (next == nullptr) {
return false; // 链表为空,无法删除
}
if (data == value) {
ListNode<T>* temp = next;
next = next->next;
delete temp;
return true;
} else {
ListNode<T>* current = this;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
ListNode<T>* temp = current->next;
current->next = current->next->next;
delete temp;
return true;
} else {
return false; // 没找到需要删除的节点
}
}
}
// 查找节点
ListNode<T>* findNode(const T& value) const {
ListNode<T>* current = this;
while (current != nullptr) {
if (current->data == value) {
return current;
}
current = current->next;
}
return nullptr; // 如果找不到,返回nullptr
}
// 打印链表
void printList() const {
ListNode<T>* current = this;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << "\n";
}
};
// 主函数测试模板类
int main() {
// 创建整型链表实例
ListNode<int> list;
// 测试插入
list.insertNode(10);
list.insertNode(20);
list.insertNode(30);
// 打印链表
list.printList(); // 输出: 10 20 30
// 测试删除
list.deleteNode(20);
list.printList(); // 输出: 10 30
// 查找节点
ListNode<int>* foundNode = list.findNode(30);
if (foundNode != nullptr) {
std::cout << "Found node with value " << foundNode->data << std::endl;
} else {
std::cout << "Node not found." << std::endl;
}
return 0;
}
```
在这个示例中,`main()`函数展示了如何使用`ListNode<int>`类,并对各种操作进行了测试。你可以根据需要替换`T`为其他类型,如`double`, `std::string`等。
阅读全文
相关推荐
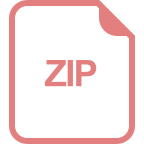
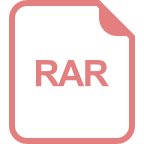





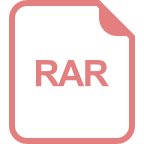
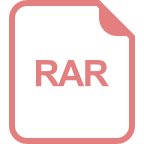
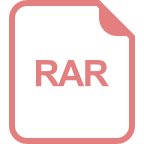





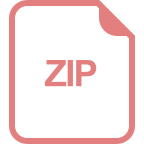
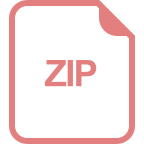
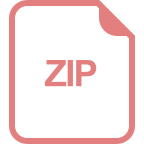
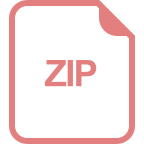