设计一个简单的单向链表类模板,并进行测试
时间: 2023-12-30 22:02:52 浏览: 74
好的,关于单向链表类模板的设计,我可以为您提供一些指导:
1. 首先需要定义一个节点类,节点包括两个成员变量:数据和指向下一个节点的指针。
2. 接着定义链表类,链表类包括一个成员变量:指向链表头节点的指针。还需要定义一些操作函数,如添加节点、删除节点、查找节点等等。
3. 最后,在测试代码中,您可以创建一个链表对象,执行各种操作函数,以确保链表类的功能正常。
以下是一个简单的单向链表类模板示例代码,希望可以帮助您:
```c++
template<typename T>
class LinkedList
{
public:
struct Node
{
T data;
Node* next;
Node(T& data) : data(data), next(nullptr) {}
};
LinkedList() : head(nullptr) {}
~LinkedList()
{
Node* current = head;
while (current != nullptr)
{
Node* next = current->next;
delete current;
current = next;
}
}
void addNode(T& data)
{
Node* newNode = new Node(data);
if (head == nullptr)
{
head = newNode;
}
else
{
Node* current = head;
while (current->next != nullptr)
{
current = current->next;
}
current->next = newNode;
}
}
bool removeNode(T& data)
{
Node* current = head;
if (head->data == data)
{
head = head->next;
delete current;
return true;
}
while (current->next != nullptr)
{
Node* next = current->next;
if (next->data == data)
{
current->next = next->next;
delete next;
return true;
}
current = next;
}
return false;
}
Node* findNode(T& data)
{
Node* current = head;
while (current != nullptr)
{
if (current->data == data)
{
return current;
}
current = current->next;
}
return nullptr;
}
private:
Node* head;
};
int main()
{
LinkedList<int> myList;
// 添加节点并测试
myList.addNode(1);
myList.addNode(2);
myList.addNode(3);
// 查找节点并测试
LinkedList<int>::Node* foundNode = myList.findNode(2);
if (foundNode != nullptr)
{
std::cout << "Found node containing data: " << foundNode->data << std::endl;
}
else
{
std::cout << "Node not found!" << std::endl;
}
// 删除节点并测试
bool success = myList.removeNode(2);
if (success)
{
std::cout << "Removed node successfully!" << std::endl;
}
else
{
std::cout << "Node not found!" << std::endl;
}
return 0;
}
```
希望这个示例能够帮助您实现一个简单的单向链表类模板。
阅读全文
相关推荐



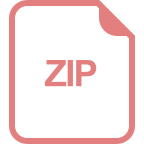






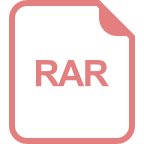
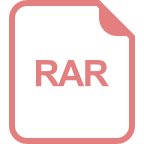
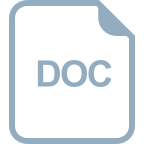