使用&读取字符串如何避免访问空指针c++
时间: 2024-11-30 22:29:36 浏览: 7
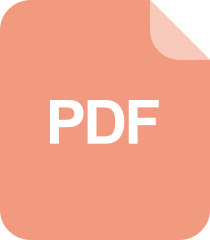
解析C++中的字符串处理函数和指针
在C++中,避免访问空指针(NULL或nullptr)特别是当处理字符串时,主要有以下几个步骤:
1. **初始化**:始终在使用字符串之前检查它是否已经被初始化。例如,如果你从用户输入或文件中读取字符串,确保分配了内存并检查返回值是否为空。
```cpp
std::string str = getUserInput();
if (!str.empty()) {
// 字符串非空,可以安全操作
}
```
2. **智能指针**:使用`std::unique_ptr<std::string>` 或 `std::shared_ptr<std::string>` 可以自动管理字符串资源,并防止空指针异常。它们会在析构函数中自动删除所指向的对象。
```cpp
std::unique_ptr<std::string> ptr(new std::string("Some string"));
if (ptr) {
// 安全地处理字符串
}
```
3. **库函数**:使用`std::getline`、`std::ifstream::getline`等标准库函数读取字符串,它们会自动处理结束标志,如换行符,并返回`std::string_view`或`std::string`,这样可以避免直接引用空对象。
4. **异常处理**:如果可能的话,在遇到空指针时捕获并处理异常,而不是让程序崩溃。
```cpp
try {
if (auto str = loadStringFromFile(fileName)) {
// 处理字符串
} else {
throw std::runtime_error("Failed to read string");
}
} catch (const std::exception &e) {
// 捕获并处理异常
}
```
总之,关键是始终确认对象存在并且非空再进行操作,同时考虑使用适当的数据结构和库函数来简化错误检查。
阅读全文
相关推荐
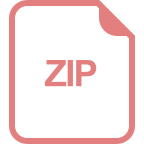
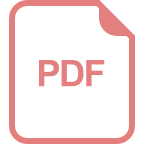
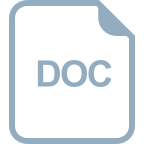
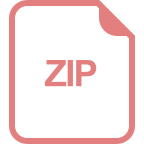
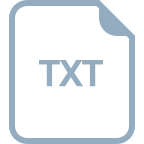
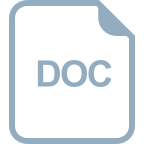
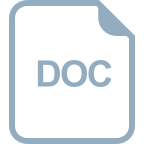
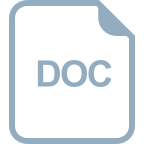
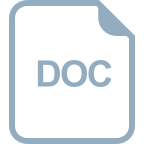
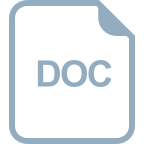
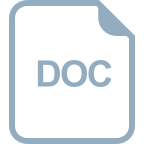
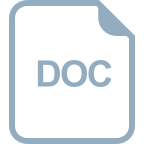
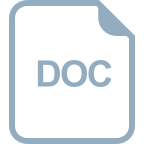





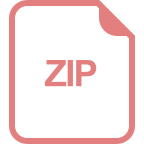