用C加加语言从键盘中输入一个字符串用一个指针指向这个字符串输出这个指针及字符串所占用的内存的大小
时间: 2024-01-22 08:18:37 浏览: 98
下面是一个示例代码,可以实现从键盘中输入一个字符串,然后用指针指向这个字符串,并输出指针及字符串所占用的内存大小:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[100];
cout << "请输入一个字符串:" << endl;
cin >> str;
char* p = str;
cout << "指针p指向的地址为:" << (void*)p << endl;
cout << "字符串所占用的内存大小为:" << strlen(str) + 1 << " 字节" << endl;
return 0;
}
```
在这个示例代码中,首先定义了一个长度为100的字符数组str,然后通过cin从键盘中读取一个字符串,并将其存储到str中。接着定义一个指针p,指向str所在的内存地址。最后,通过strlen函数计算出字符串的长度,并加上1(因为字符串末尾还有一个空字符'\0'),即可得到字符串所占用的内存大小。使用(void*)p强制转换为void指针类型,是为了能够输出指针指向的内存地址。
相关问题
用C加加语言采用指针输入字符串并在屏幕上打印
下面是一个用 C++ 语言编写的函数,该函数采用指针输入字符串并在屏幕上打印:
```cpp
#include <iostream>
using namespace std;
void printString(char *str) {
while (*str != '\0') {
cout << *str;
str++;
}
cout << endl;
}
int main() {
char str[] = "Hello, World!";
printString(str);
return 0;
}
```
在这个函数中,我们使用了一个指针来迭代字符串中的每个字符。while 循环在字符串的末尾遇到空字符时停止,然后我们打印出整个字符串并在最后添加一个换行符。
在主函数中,我们声明了一个字符数组 str 并将其初始化为 "Hello, World!"。然后,我们调用 printString 函数,并将 str 的地址作为参数传递给它。最后,我们在主函数中返回 0。
输入3个字符串,按由小到大的顺序输出,用C加加,并且使用指针
```c++
#include<iostream>
#include<string>
using namespace std;
int main() {
string str1, str2, str3;
cout << "请输入3个字符串:" << endl;
cin >> str1 >> str2 >> str3;
string *p1 = &str1;
string *p2 = &str2;
string *p3 = &str3;
if (*p1 > *p2) {
string *temp = p1;
p1 = p2;
p2 = temp;
}
if (*p1 > *p3) {
string *temp = p1;
p1 = p3;
p3 = temp;
}
if (*p2 > *p3) {
string *temp = p2;
p2 = p3;
p3 = temp;
}
cout << "按由小到大的顺序输出:" << endl;
cout << *p1 << " " << *p2 << " " << *p3 << endl;
return 0;
}
```
阅读全文
相关推荐
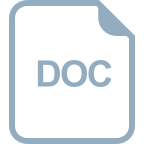
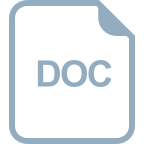
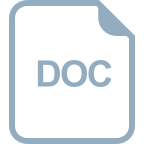


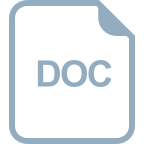






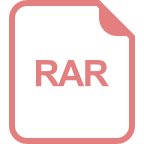
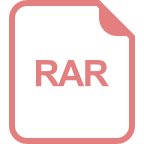
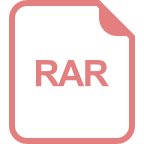
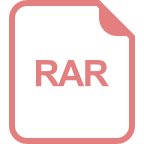
