做事应该有始有终吧,做一个高级版。并给予他能够从图片中随便选取一个人脸,并标注这个人脸它可以在点击拍照后并随机抽取。
时间: 2024-12-15 08:19:37 浏览: 5
创建一个更高级的脸部抽签系统,你可以使用Python的深度学习库如`cv2`进行图像处理,`dlib`进行面部检测,以及`numpy`和`random`进行随机选择。这里是一个相对复杂的版本:
```python
import cv2
import numpy as np
import dlib
from PIL import Image
# 下载预训练模型(需要事先安装dlib)
# 下载地址:https://github.com/davisking/dlib-models/raw/master/mmod_human_face_detector.dat.bz2
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor('shape_predictor_68_face_landmarks.dat')
def detect_faces(image_path):
gray_img = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE)
faces = detector(gray_img)
return faces
def draw_bounding_boxes(image, faces):
for face in faces:
x, y, w, h = face.left(), face.top(), face.width(), face.height()
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
landmarks = predictor(gray_img, face)
for n in range(68):
cv2.circle(image, (landmarks.part(n).x, landmarks.part(n).y), 2, (0, 255, 0), -1)
return image
def select_random_face(faces):
return random.choice(faces)
def main():
# 初始化一个空列表存储选定的人脸
selected_faces = []
while True:
# 用户输入或者摄像头捕捉
user_input = input("请输入图片路径(按q退出):")
if user_input.lower() == 'q':
break
image = cv2.imread(user_input)
faces_in_image = detect_faces(image_path=user_input)
for face in faces_in_image:
# 绘制人脸框并获取关键点
annotated_image = draw_bounding_boxes(image.copy(), [face])
# 保存标注后的图片
cv2.imwrite('annotated_' + user_input, annotated_image)
# 添加到选择列表
selected_faces.append(face)
if len(selected_faces) > 0:
random_face = select_random_face(selected_faces)
print("随机抽取的人脸:", random_face)
else:
print("未检测到任何人脸,请检查输入或再次尝试.")
if __name__ == "__main__":
main()
```
在这个高级版中,用户可以选择拍照或输入图片路径,系统会自动检测并标注每个人脸,然后在下一次循环中随机选择一张。注意这只是一个基础示例,实际应用中可能还需要处理异常和用户交互的细节。
阅读全文
相关推荐
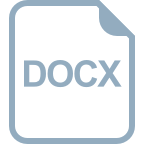
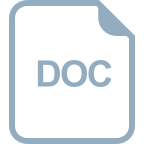
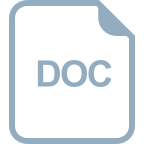
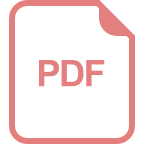
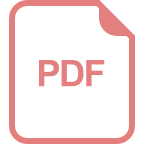
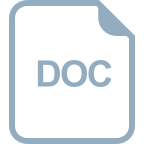
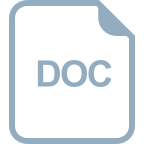
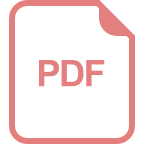
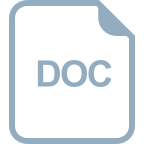
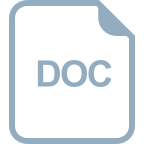
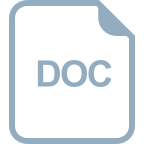
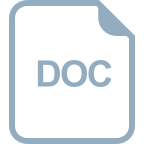
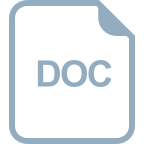
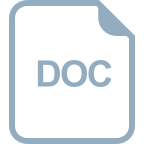
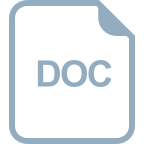
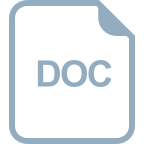
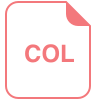

