Java 实现小球碰撞的代码
时间: 2024-10-13 10:04:41 浏览: 15
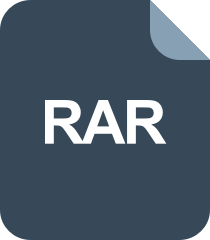
Java实现的碰撞检测代码-测试小球碰撞边缘.rar
在Java中实现小球碰撞的基本步骤通常涉及到游戏编程中的物理模拟。这里提供一个简单的二维圆形小球碰撞检测的基本示例代码:
```java
public class Ball {
private double x, y; // 小球的位置坐标
private double radius; // 小球半径
private boolean isColliding; // 碰撞标志
public Ball(double x, double y, double radius) {
this.x = x;
this.y = y;
this.radius = radius;
this.isColliding = false;
}
// 检测两个小球之间的碰撞
public boolean checkCollision(Ball otherBall) {
double dx = this.x - otherBall.x;
double dy = this.y - otherBall.y;
double distance = Math.sqrt(dx * dx + dy * dy);
if (distance < this.radius + otherBall.radius) { // 如果距离小于两者半径之和,认为有碰撞
isColliding = true;
return true;
} else {
isColliding = false;
return false;
}
}
public void setX(double newX) {
if (!isColliding && Math.abs(newX - this.x) > radius + otherBall.radius) {
this.x = newX;
} // 添加边界处理,避免碰撞时改变位置
}
public void setY(double newY) {
// 同理,添加边界处理
if (!isColliding && Math.abs(newY - this.y) > radius + otherBall.radius) {
this.y = newY;
}
}
// 返回是否正在碰撞
public boolean isCollides() {
return isColliding;
}
}
// 游戏循环中更新并检查碰撞
Ball ball1 = new Ball(0, 0, 5);
Ball ball2 = new Ball(50, 50, 5);
while (true) {
// 更新每个球的位置
ball1.setX(ball1.getX() + 1); // 假设小球每次向右移动
ball1.setY(ball1.getY() + 1);
ball2.setX(ball2.getX() - 1); // 另一个小球每次向左移动
ball2.setY(ball2.getY() - 1);
// 检查碰撞
if (ball1.checkCollision(ball2)) {
System.out.println("小球发生了碰撞!");
}
}
```
阅读全文
相关推荐
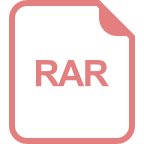
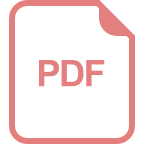
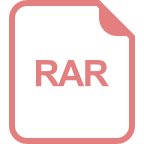
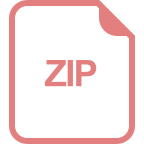
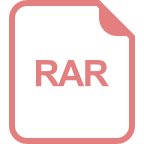
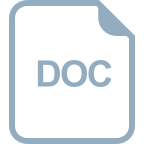
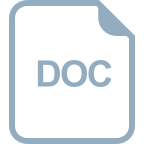
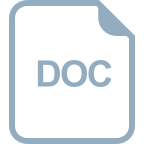
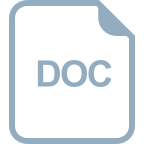



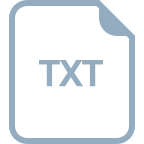
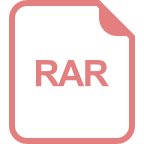
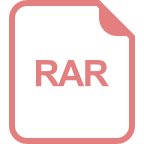

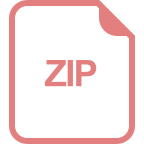