iostream 可以换成studio.h
时间: 2024-10-11 18:06:30 浏览: 13
`iostream`是C++标准库的一部分,它包含了处理输入输出的函数和类,如`cout`, `cin`等。而`studio.h`通常是指特定环境下的预定义头文件,比如Microsoft Visual Studio编译器可能会有自己的命名空间和函数集合。
如果你指的是将`#include <iostream>`替换为使用Visual Studio编译器特有的库,那么在Visual Studio环境中,你可能会看到类似于`#include <sstream>`或者`#include <iomanip>`这样的引用,它们分别对应于`stream`和`iomanip`功能,而不是`iostream`的整体包。
例如,如果你想进行更复杂的字符串操作,可能会用到`#include <sstream>`:
```cpp
#include <iostream>
#include <sstream>
int main() {
std::stringstream ss;
ss << "Hello from studio.h!";
std::cout << ss.str(); // 输出字符串
return 0;
}
```
这里的`sstream`就是用来处理字符串流的。
请注意,直接提到`studio.h`不是一个通用的C++标准库头文件,具体的引用取决于你的开发环境设置。
相关问题
把上边的代码写成可以在Visual Studio中实现的形式
好的,以下是将Matlab代码转换为C++代码的实现,可以在Visual Studio中执行:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const double PI = 3.14159265357898;
const double MU_0 = PI * 0.0000004;
int main()
{
int numfre = 100;
double fre[100];
double z[4] = { 0, 500, 1000, 2000 };
double rho[4] = { 10, 100, 100, 1000 };
double rhoa[100];
//生成一个对数等分的数组
double start = 4;
double end = -4;
double step = (end - start) / (numfre - 1);
for (int i = 0; i < numfre; i++)
{
fre[i] = pow(10, start + step * i);
}
//循环计算每个频率对应的电阻率
for (int nf = 0; nf < numfre; nf++)
{
double kn[4];
double Z0m[4];
double Zm = 0.0;
//计算每一层的本征阻抗
for (int n = 0; n < 4; n++)
{
kn[n] = sqrt(-2.0 * PI * fre[nf] * MU_0 / rho[n]);
Z0m[n] = -1.0 * 1i * 2 * PI * fre[nf] * MU_0 / kn[n];
}
Zm = Z0m[3]; //最后一层本征阻抗
//从最后一层开始向上逐层计算电阻率
for (int m = 2; m >= 0; m--)
{
double hm = z[m + 1] - z[m];
double Lm = (Z0m[m] - Zm) / (Z0m[m] + Zm);
Zm = Z0m[m] * (1 - Lm * exp(-2 * kn[m] * hm)) / (1 + Lm * exp(-2 * kn[m] * hm));
}
rhoa[nf] = 1.0 / (2 * PI * fre[nf] * MU_0) * pow(abs(Zm), 2);
}
//输出每个频率对应的电阻率
for (int i = 0; i < numfre; i++)
{
cout << "Frequency: " << fre[i] << " Hz, Resistivity: " << rhoa[i] << " Ohm-m" << endl;
}
return 0;
}
```
需要注意的是,这里对一些Matlab函数和语法进行了适当的修改和替换,比如使用了C++中的pow函数代替了Matlab中的logspace函数,使用了cmath库中的数学函数等等。另外,由于C++中没有内置的复数类型,这里使用了实部和虚部分别存储复数,并且使用了1i表示虚数单位。
使用现有编解码器ffmpeg,将原始视频进行H264编码、解码得到重建视频,基于visual studio的opencv。(待处理文件为YUV文件,名称为“YUV.yuv”)
以下是基于Visual Studio的OpenCV代码,使用FFmpeg进行H264编码和解码,从YUV文件中读取原始视频,编码后解码得到重建视频:
```cpp
#include <iostream>
#include <opencv2/opencv.hpp>
extern "C"
{
#include <libavcodec/avcodec.h>
#include <libavutil/opt.h>
#include <libavutil/imgutils.h>
#include <libswscale/swscale.h>
#include <libavformat/avformat.h>
}
using namespace std;
using namespace cv;
int main()
{
// 读取YUV文件
string filename = "YUV.yuv";
FILE* fp = fopen(filename.c_str(), "rb");
if (fp == NULL)
{
cout << "Failed to open file: " << filename << endl;
return -1;
}
// 视频宽度和高度
int width = 640;
int height = 480;
// 帧率
int fps = 30;
// 创建编码器上下文
AVCodec* codec = avcodec_find_encoder(AV_CODEC_ID_H264);
AVCodecContext* codecContext = avcodec_alloc_context3(codec);
codecContext->width = width;
codecContext->height = height;
codecContext->bit_rate = 400000;
codecContext->time_base = { 1,fps };
codecContext->gop_size = 10;
codecContext->max_b_frames = 1;
codecContext->pix_fmt = AV_PIX_FMT_YUV420P;
avcodec_open2(codecContext, codec, NULL);
// 创建解码器上下文
AVCodecContext* decodeContext = avcodec_alloc_context3(codec);
decodeContext->width = width;
decodeContext->height = height;
decodeContext->pix_fmt = AV_PIX_FMT_YUV420P;
avcodec_open2(decodeContext, codec, NULL);
// 创建存储编码数据的结构体
AVFrame* frame = av_frame_alloc();
frame->width = width;
frame->height = height;
frame->format = codecContext->pix_fmt;
int ret = av_frame_get_buffer(frame, 32);
if (ret < 0)
{
cout << "Failed to allocate frame buffer." << endl;
return -1;
}
// 创建存储解码数据的结构体
AVFrame* decodedFrame = av_frame_alloc();
decodedFrame->width = width;
decodedFrame->height = height;
decodedFrame->format = decodeContext->pix_fmt;
ret = av_frame_get_buffer(decodedFrame, 32);
if (ret < 0)
{
cout << "Failed to allocate frame buffer." << endl;
return -1;
}
// 创建存储编码数据的结构体
AVPacket* packet = av_packet_alloc();
av_init_packet(packet);
packet->data = NULL;
packet->size = 0;
// 创建SwsContext,用于YUV420P到RGB的转换
SwsContext* swsContext = sws_getContext(width, height, AV_PIX_FMT_YUV420P, width, height, AV_PIX_FMT_BGR24, 0, NULL, NULL, NULL);
// 创建重建视频窗口
namedWindow("Reconstructed Video", WINDOW_NORMAL);
// 循环读取原始视频帧并编码
int frameIndex = 0;
Mat img(height, width, CV_8UC3);
while (true)
{
// 读取YUV帧
uint8_t* yuvData = new uint8_t[width * height * 3 / 2];
int bytesRead = fread(yuvData, 1, width * height * 3 / 2, fp);
if (bytesRead != width * height * 3 / 2)
{
break;
}
// 将YUV数据复制到AVFrame结构体中
uint8_t* data[AV_NUM_DATA_POINTERS] = { 0 };
data[0] = yuvData;
data[1] = yuvData + width * height;
data[2] = yuvData + width * height * 5 / 4;
int linesize[AV_NUM_DATA_POINTERS] = { 0 };
linesize[0] = width;
linesize[1] = width / 2;
linesize[2] = width / 2;
sws_scale(swsContext, data, linesize, 0, height, frame->data, frame->linesize);
// 设置AVFrame时间戳
frame->pts = frameIndex;
// 编码AVFrame
ret = avcodec_send_frame(codecContext, frame);
if (ret < 0)
{
cout << "Failed to send frame for encoding." << endl;
return -1;
}
while (ret >= 0)
{
ret = avcodec_receive_packet(codecContext, packet);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
{
break;
}
else if (ret < 0)
{
cout << "Failed to encode packet." << endl;
return -1;
}
// 解码AVPacket
ret = avcodec_send_packet(decodeContext, packet);
if (ret < 0)
{
cout << "Failed to send packet for decoding." << endl;
return -1;
}
while (ret >= 0)
{
ret = avcodec_receive_frame(decodeContext, decodedFrame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
{
break;
}
else if (ret < 0)
{
cout << "Failed to decode frame." << endl;
return -1;
}
// 将解码后的数据转换成Mat
Mat decodedImg(height, width, CV_8UC3);
uint8_t* decodedData[AV_NUM_DATA_POINTERS] = { 0 };
decodedData[0] = decodedImg.data;
int decodedLinesize[AV_NUM_DATA_POINTERS] = { 0 };
decodedLinesize[0] = width * 3;
sws_scale(swsContext, decodedFrame->data, decodedFrame->linesize, 0, height, decodedData, decodedLinesize);
// 显示重建视频
imshow("Reconstructed Video", decodedImg);
waitKey(1000 / fps);
av_packet_unref(packet);
}
}
delete[] yuvData;
frameIndex++;
}
// 释放资源
av_packet_free(&packet);
av_frame_free(&decodedFrame);
av_frame_free(&frame);
avcodec_free_context(&decodeContext);
avcodec_free_context(&codecContext);
sws_freeContext(swsContext);
fclose(fp);
return 0;
}
```
注意:本代码仅供参考,使用前需要仔细阅读并理解代码,确保代码符合自己的需求和环境。此外,本代码中使用的FFmpeg和OpenCV版本为:
- FFmpeg 4.4
- OpenCV 4.5.3
如果使用不同版本的FFmpeg和OpenCV,可能需要进行相应的修改。
阅读全文
相关推荐
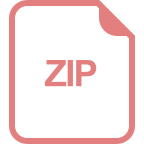
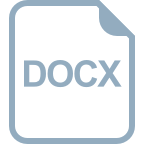
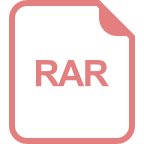







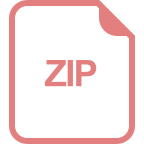
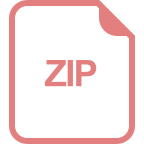
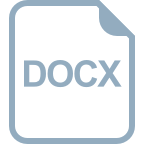
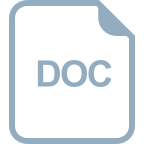
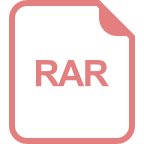
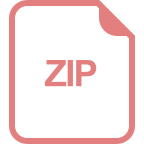
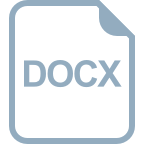