编写程序读入文档并分词c++代码编写
时间: 2024-10-09 09:07:44 浏览: 45
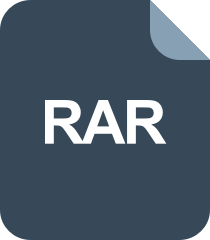
Excel_VBA.rar_Excel VBA_excel代码编写
在C++中,处理文本文件并进行分词通常涉及到文件I/O操作以及字符串处理。这里是一个简单的示例,展示了如何使用`fstream`库来读取文件,然后使用内置的`std::istringstream`和`std::string`来逐行分词:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <string>
// 这里可以使用更复杂的分词库如jieba,但这里是简单示例
std::vector<std::string> split(const std::string &s, char delimiter) {
std::vector<std::string> tokens;
size_t pos = 0;
std::string token;
while ((pos = s.find(delimiter)) != std::string::npos) {
token = s.substr(0, pos);
if (!token.empty()) {
tokens.push_back(token);
}
s.erase(0, pos + 1); // erase found delimiter
}
if (s.length() > 0) {
tokens.push_back(s);
}
return tokens;
}
int main() {
std::ifstream file("your_file.txt"); // 替换为你要读取的文件路径
if (!file.is_open()) {
std::cerr << "Failed to open the file" << std::endl;
return 1;
}
std::string line;
while (getline(file, line)) { // 逐行读取文件
std::istringstream iss(line);
std::vector<std::string> words;
for (std::string word; iss >> word; ) { // 分词
words.push_back(word);
}
// 打印或进一步处理分词结果
for (const auto& w : words) {
std::cout << w << " ";
}
std::cout << std::endl;
}
file.close();
return 0;
}
```
这个代码片段假设每行就是一个完整的句子,通过空格分词。如果你需要更复杂的情况(比如标点符号、停用词等),可能需要使用正则表达式或其他专门的文本处理库。
阅读全文
相关推荐
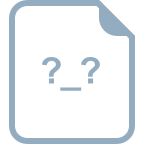
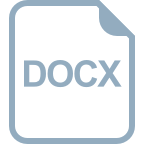
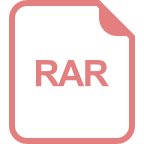
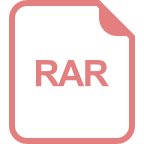
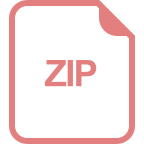
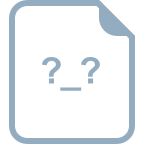
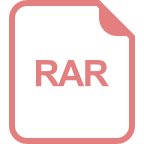
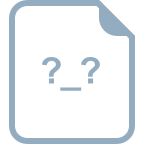
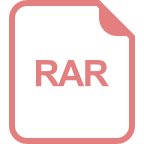
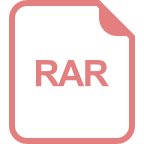
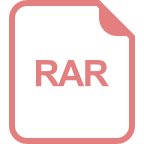
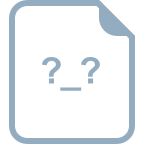
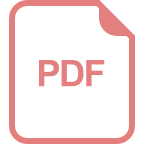
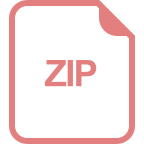
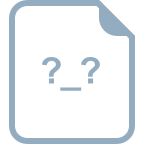