from flask import Blueprint blog_bp = Blueprint('blog',__name__) 代码解释
时间: 2024-04-19 10:29:21 浏览: 156
这段代码是使用Flask框架创建一个蓝图(Blueprint)对象,用于组织和管理与博客相关的路由和视图函数。下面是代码的解释:
- `from flask import Blueprint`: 导入Flask框架中的Blueprint类,用于创建蓝图对象。
- `blog_bp = Blueprint('blog',__name__)`: 创建一个名为`blog`的蓝图对象。`'blog'`是蓝图的名称,`__name__`表示当前模块的名称,用于指定蓝图所在的包或模块。
通过使用蓝图对象,可以将相关的路由和视图函数组织在一起,并将它们与应用程序进行关联。在这个例子中,`blog_bp`是一个名为`blog`的蓝图对象。
使用蓝图可以有效地组织和管理大型应用程序的路由和视图函数,使代码更加模块化和可维护。蓝图还可以实现模块之间的解耦,提高应用程序的可扩展性。
相关问题
user_bp1 = Blueprint('user',__name__,url_prefix='/user') NameError: name 'Blueprint' is not defined
This error message indicates that the `Blueprint` object has not been imported in your Python script.
To fix this error, you need to import the `Blueprint` object from the `flask` package by adding the following import statement at the top of your Python script:
```python
from flask import Blueprint
```
Then you can create a new blueprint object as follows:
```python
user_bp1 = Blueprint('user', __name__, url_prefix='/user')
```
Make sure that the `flask` package is installed in your Python environment. You can install it using pip:
```
pip install flask
```
After installing the `flask` package and importing the `Blueprint` object, the `NameError` should be resolved.
用python写一段代码,flask的blueprint 异步发送带附件的邮件
以下是使用 Python 和 Flask 的 Blueprint 异步发送带附件的邮件的代码示例:
```python
from flask import Blueprint, current_app, jsonify, request
from flask_mail import Message, Mail
import threading
mail_bp = Blueprint('mail', __name__)
mail = Mail()
def send_async_email(app, msg):
with app.app_context():
mail.send(msg)
@mail_bp.route('/send_mail', methods=['POST'])
def send_mail():
data = request.get_json()
recipient = data.get('recipient')
subject = data.get('subject')
body = data.get('body')
attachment = data.get('attachment')
msg = Message(subject=subject, recipients=[recipient])
msg.body = body
with current_app.open_resource(attachment) as f:
msg.attach(attachment, 'application/octet-stream', f.read())
thread = threading.Thread(target=send_async_email, args=(current_app._get_current_object(), msg))
thread.start()
return jsonify({'message': 'Email sent successfully.'}), 200
```
请注意,此代码示例仅供参考。在实际使用中,您需要根据您的具体需求进行修改和调整。
阅读全文
相关推荐
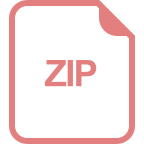
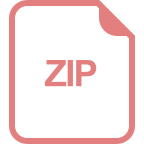
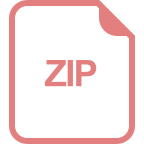
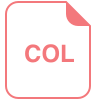

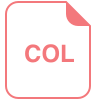
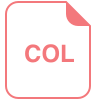









