ChitGPT 的 API如何集成进java
时间: 2024-06-09 18:12:24 浏览: 84
要将ChitGPT的API集成到Java中,您需要使用Java HTTP客户端库,例如Apache的HttpClient或OkHttp。然后,您可以使用ChitGPT的API端点来向服务器发送POST请求,并获得AI的响应结果。您可以使用JSON库来解析响应并将其集成到您的Java应用程序中。
以下是一个示例代码片段,使用Apache HttpClient发送请求并解析JSON响应:
```java
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.json.JSONObject;
public class ChitGPTClient {
private final String API_ENDPOINT = "https://api.chitgpt.com/gpt-chatbot/v1/chat";
public String getChitGPTResponse(String userInput) throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(API_ENDPOINT);
JSONObject json = new JSONObject();
json.put("message", userInput);
StringEntity entity = new StringEntity(json.toString());
httpPost.setEntity(entity);
httpPost.setHeader("Accept", "application/json");
httpPost.setHeader("Content-type", "application/json");
CloseableHttpResponse response = httpClient.execute(httpPost);
String responseString = EntityUtils.toString(response.getEntity(), "UTF-8");
JSONObject responseObject = new JSONObject(responseString);
String botResponse = responseObject.getString("response");
return botResponse;
}
}
```
您可以使用以下方式调用该方法:
```java
String userInput = "Hello, ChitGPT!";
ChitGPTClient client = new ChitGPTClient();
String botResponse = client.getChitGPTResponse(userInput);
System.out.println(botResponse);
```
请注意,您需要在代码中设置适当的身份验证令牌或密钥,以便使用ChitGPT的API。
阅读全文
相关推荐
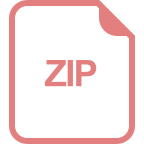
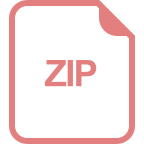















