凯撒密码python作业
时间: 2023-10-14 07:06:43 浏览: 45
以下是一个简单的凯撒密码 Python 程序:
```python
def caesar_cipher(text, shift):
cipher = ""
for char in text:
if char.isalpha():
cipher += chr((ord(char) + shift - 65) % 26 + 65)
else:
cipher += char
return cipher
text = input("请输入明文:")
shift = int(input("请输入偏移量:"))
print("凯撒密码加密结果:", caesar_cipher(text, shift))
```
这个程序会提示用户输入需要加密的明文和偏移量,然后输出加密后的密文。
程序的核心是 `caesar_cipher()` 函数,它接受两个参数:需要加密的明文和偏移量。函数会遍历明文中的每个字符,对于字母字符,将它的 ASCII 码加上偏移量,再对 26 取模,最后加上 65(即大写字母 A 的 ASCII 码)得到密文中对应的 ASCII 码。对于非字母字符,直接将它添加到密文中。最后返回密文。
注意,这个程序只支持大写字母的加密,如果需要支持小写字母和其他符号,需要进行相应的修改。
相关问题
凯撒密码Python
凯撒密码是一种简单的替换密码,它是通过将字母按照一定的偏移量进行替换来加密和解密文本的。具体来说,凯撒密码将字母表中的每个字母都向后(或向前)移动固定的位置,这个移动的位置就是密钥。
下面是一个用Python实现凯撒密码的例子:
```python
def caesar_cipher(text, key):
encrypted_text = ""
for char in text:
if char.isalpha():
if char.isupper():
encrypted_text += chr((ord(char) - 65 + key) % 26 + 65)
else:
encrypted_text += chr((ord(char) - 97 + key) % 26 + 97)
else:
encrypted_text += char
return encrypted_text
# 加密示例
plaintext = "Hello, World!"
key = 3
ciphertext = caesar_cipher(plaintext, key)
print("加密后的文本:", ciphertext)
# 解密示例
decrypted_text = caesar_cipher(ciphertext, -key)
print("解密后的文本:", decrypted_text)
```
在上面的代码中,`caesar_cipher`函数接受两个参数:`text`表示要加密或解密的文本,`key`表示移动的偏移量。函数首先遍历文本中的每个字符,如果是字母,则根据大小写分别进行移位操作,并将结果拼接到`encrypted_text`字符串中。最后返回加密或解密后的文本。
请注意,这只是凯撒密码的一种简单实现,它只能对字母进行加密和解密,对于其他字符(如数字、标点符号等)会保持不变。此外,凯撒密码的安全性非常低,容易被破解,因此在实际应用中很少使用。
凯撒密码 python
凯撒密码是一种简单且广为人知的加密技术,它是一种替换加密的技术,将明文中的所有字母按照字母表的顺序向后偏移固定数目后变成密文。在Python中解决凯撒密码可以使用以下步骤:
1. 首先,定义一个函数来实现凯撒密码的加密和解密操作。该函数需要接收两个参数:明文和偏移量。偏移量可以是正数(向后偏移)或负数(向前偏移)。
2. 在函数内部,使用一个循环来遍历明文中的每个字符。对于每个字符,根据其在字母表中的位置进行偏移。可以使用内置的ord()函数将字符转换为ASCII码,并使用chr()函数将偏移后的ASCII码转换回字符。
3. 注意处理大写字母和小写字母的情况。可以使用内置的isupper()函数和islower()函数来判断字符是否为大写或小写,并根据情况进行相应的处理。
4. 最后,返回加密或解密后的结果。
以下是一个示例的凯撒密码加密和解密函数的实现:
```python
def caesar_cipher(text, shift):
result = ""
for char in text:
if char.isalpha():
if char.isupper():
result += chr((ord(char) - 65 + shift) % 26 + 65)
else:
result += chr((ord(char) - 97 + shift) % 26 + 97)
else:
result += char
return result
```
使用该函数,你可以将明文加密为凯撒密码,也可以将凯撒密码解密回明文。例如,使用偏移量3对明文"hello"进行加密:
```python
plaintext = "hello"
shift = 3
ciphertext = caesar_cipher(plaintext, shift)
print(ciphertext) # 输出:"khoor"
```
相关推荐
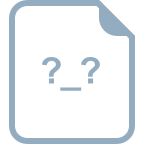
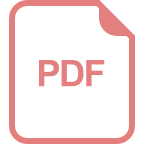











