凯撒密码python编程移3个
时间: 2024-06-01 16:06:26 浏览: 111
凯撒密码是一种古老的加密算法,将明文中的每个字母向后移动固定数量的位置,从而得到密文。移动的位置数被称为密钥。Python可以很方便地实现凯撒密码的加密和解密。
以下是一个简单的凯撒密码加密程序,该程序将明文中的每个字母向后移动三个位置。
```python
def caesar_encrypt(text):
result = ""
# 遍历明文中的每个字符
for i in range(len(text)):
char = text[i]
# 如果字符是大写字母
if char.isupper():
result += chr((ord(char) + 3 - 65) % 26 + 65)
# 如果字符是小写字母
elif char.islower():
result += chr((ord(char) + 3 - 97) % 26 + 97)
else:
result += char
return result
text = input("请输入要加密的明文:")
print("加密结果为:" + caesar_encrypt(text))
```
你只需要在命令行输入要加密的明文,程序会输出加密后的结果。如果要解密,只需要将密文中的每个字母向前移动三个位置即可。
相关问题
凯撒密码python编程
凯撒密码是一种简单的加密算法,通过将字母按照一定的偏移量进行替换来实现加密和解密。在Python中实现凯撒密码可以通过定义一个字母表,并利用其索引进行偏移计算。根据引用中的代码示例,我们可以给出以下Python代码来实现凯撒密码的加密和解密功能:
```python
import string
upper_list = string.ascii_uppercase
lower_list = string.ascii_lowercase
def encryption(source):
offset = 5
result = ''
for c in source:
if c in lower_list:
index = (lower_list.index(c) + offset) % len(lower_list)
result += lower_list[index]
elif c in upper_list:
index = (upper_list.index(c) + offset) % len(lower_list)
result += upper_list[index]
return result
def decryption(result):
offset = 5
source = ''
for c in result:
if c in lower_list:
index = (lower_list.index(c) - offset) % len(lower_list)
source += lower_list[index]
elif c in upper_list:
index = (upper_list.index(c) - offset) % len(lower_list)
source += upper_list[index]
return source
if __name__ == '__main__':
source = "JiaYou"
encrypted_result = encryption(source)
decrypted_result = decryption(encrypted_result)
print(encrypted_result)
print(decrypted_result)
```
以上代码中,`encryption`函数用于将源字符串进行加密,`decryption`函数用于将加密结果进行解密。在`if __name__ == '__main__'`部分,我们可以看到使用示例,输入"JiaYou"字符串进行加密后得到"OnfDtz",再对加密结果进行解密得到原始字符串"JiaYou"。
凯撒密码python编程解密
凯撒密码是一种简单的替换式加密方法,通过将明文字母按照固定偏移量进行位移来达到加密的目的。在Python中解密凯撒密码,通常涉及以下步骤:
1. 确定偏移量:这取决于加密时使用的具体的偏移值,可能是用户输入、硬编码的固定值或从密文中推断。
2. 创建一个字母表:原始的是大写或小写字母表(A-Z或a-z),以及可能包含数字和特殊字符的扩展字母表,如果加密时包含了这些。
3. 解密函数:编写一个函数,接收加密后的字符串和偏移量作为输入,对每个字符应用相反的偏移,然后返回解密后的字符串。
下面是一个简单的Python解密函数示例(只处理英文大写字母):
```python
def caesar_cipher_decrypt(ciphertext, shift):
alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
decrypted_text = ""
for char in ciphertext:
if char.isalpha():
is_upper = char.isupper()
index = (alphabet.index(char) - shift) % len(alphabet)
if is_upper:
decrypted_text += alphabet[index].upper()
else:
decrypted_text += alphabet[index].lower()
else:
decrypted_text += char
return decrypted_text
# 示例
ciphertext = "KHOOR ZRUOG" # 加密后的文本,假设偏移量为3
shift = 3
decrypted_text = caesar_cipher_decrypt(ciphertext, shift)
print(f"原加密文本: {ciphertext}\n解密后文本: {decrypted_text}")
```
阅读全文
相关推荐
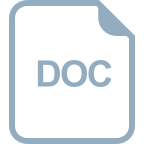
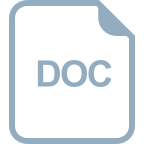
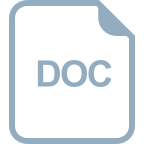
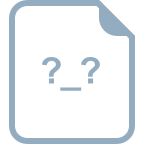






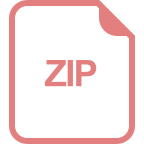
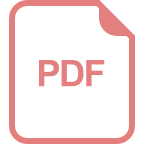
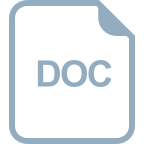



