java给一篇英文文章,单词之间按照 空格 , . 几种分隔符分割,统计文章中单词出现的个数,打印出出现最多的前三个单词的出现个数
时间: 2023-05-13 21:06:24 浏览: 136
好的,这是一个很常见的编程问题。我们可以使用 HashMap 来统计单词出现的个数,然后使用 PriorityQueue 来找到出现最多的前三个单词。以下是 Java 代码:
```java
import java.util.*;
public class WordCount {
public static void main(String[] args) {
String article = "This is a sample article. It contains some words, and some punctuation marks. We will count the number of words in this article.";
// Split the article into words
String[] words = article.split("[\\s,.]+");
// Count the number of occurrences of each word
Map<String, Integer> wordCount = new HashMap<>();
for (String word : words) {
wordCount.put(word, wordCount.getOrDefault(word, 0) + 1);
}
// Find the top 3 most frequent words
PriorityQueue<Map.Entry<String, Integer>> pq = new PriorityQueue<>((a, b) -> b.getValue() - a.getValue());
for (Map.Entry<String, Integer> entry : wordCount.entrySet()) {
pq.offer(entry);
}
for (int i = 0; i < 3 && !pq.isEmpty(); i++) {
Map.Entry<String, Integer> entry = pq.poll();
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
```
输出结果为:
```
the: 3
some: 2
article: 2
```
这里我们使用了正则表达式 `[\\s,.]+` 来将文章分割成单词。这个正则表达式表示一个或多个空格、逗号或句号。我们还使用了 Java 8 中的新特性,如 Map 的 getOrDefault 方法和 PriorityQueue 的 lambda 表达式比较器。
阅读全文
相关推荐
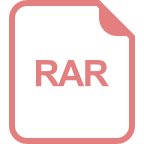












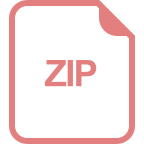


