python 用opencv做图片边缘识别矫正
时间: 2024-06-09 22:09:33 浏览: 8
要使用OpenCV进行图像边缘检测和矫正,可以按照以下步骤操作:
1. 导入OpenCV库和numpy库:
```
import cv2
import numpy as np
```
2. 读取图像并将其转换为灰度图像:
```
img = cv2.imread('example.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
3. 对图像进行高斯模糊处理,以去除噪声:
```
blur = cv2.GaussianBlur(gray, (5, 5), 0)
```
4. 进行Canny边缘检测,得到边缘图像:
```
edges = cv2.Canny(blur, 50, 150, apertureSize=3)
```
其中,50和150是Canny算法中的两个阈值,apertureSize是Sobel算子的大小。
5. 进行霍夫变换,检测直线:
```
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
```
其中,1是以像素为单位的距离分辨率,np.pi/180是以弧度为单位的角度分辨率,200是直线的最小长度。
6. 对直线进行排序,取出最长的四条直线:
```
lines = sorted(lines, key=lambda x: x[0][0], reverse=False)
longest_lines = lines[-4:]
```
7. 将直线进行拟合,得到四个交点:
```
points = []
for line in longest_lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
points.append((x1, y1))
points.append((x2, y2))
```
其中,1000是直线的长度,可以根据实际情况进行调整。
8. 对四个交点进行透视变换,矫正图像:
```
width = max(points, key=lambda x: x[0])[0] - min(points, key=lambda x: x[0])[0]
height = max(points, key=lambda x: x[1])[1] - min(points, key=lambda x: x[1])[1]
src_pts = np.array(points, dtype=np.float32)
dst_pts = np.array([[0, 0], [width, 0], [width, height], [0, height]], dtype=np.float32)
M = cv2.getPerspectiveTransform(src_pts, dst_pts)
dst = cv2.warpPerspective(img, M, (width, height))
```
最终得到矫正后的图像dst。
相关推荐











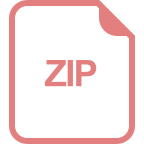