python提取图片中表格数据的思路和代码
时间: 2023-10-01 13:09:59 浏览: 394
提取图片中的表格数据可以采用以下思路:
1. 图像预处理:将彩色图像转为灰度图像,并进行二值化处理,使表格线条更加明显。
2. 边缘检测:使用Canny算法进行边缘检测,找到表格的边缘。
3. 轮廓检测:使用OpenCV的findContours函数找到边缘的所有轮廓。
4. 轮廓筛选:根据轮廓的周长、面积等特征,筛选出可能是表格的轮廓。
5. 表格校正:使用透视变换将表格矫正为矩形。
6. 单元格分割:根据表格的行列数,将表格分割为单元格。
7. OCR识别:对每个单元格进行OCR文字识别,得到表格数据。
下面是一个简单的Python代码示例,仅供参考:
```python
import cv2
import pytesseract
# 读取图像
img = cv2.imread('table.jpg')
# 图像预处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV | cv2.THRESH_OTSU)[1]
# 边缘检测
edges = cv2.Canny(thresh, 50, 150, apertureSize=3)
# 轮廓检测
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 轮廓筛选
table_contours = []
for c in contours:
x, y, w, h = cv2.boundingRect(c)
if w > 50 and h > 50 and abs(w - h) < 30:
table_contours.append(c)
# 表格校正
if len(table_contours) > 0:
rect = cv2.minAreaRect(table_contours[0])
box = cv2.boxPoints(rect)
box = box.astype(int)
cv2.drawContours(img, [box], 0, (0, 0, 255), 2)
warp = cv2.getPerspectiveTransform(box, ((0, 0), (500, 0), (500, 500), (0, 500)))
warped = cv2.warpPerspective(img, warp, (500, 500))
# 单元格分割
rows = 10
cols = 10
cell_w = warped.shape[1] // cols
cell_h = warped.shape[0] // rows
for i in range(rows):
for j in range(cols):
x1, y1 = j * cell_w, i * cell_h
x2, y2 = (j + 1) * cell_w, (i + 1) * cell_h
cell = warped[y1:y2, x1:x2]
cv2.imwrite(f'cell_{i}_{j}.jpg', cell)
# OCR识别
pytesseract.pytesseract.tesseract_cmd = r'C:\Program Files\Tesseract-OCR\tesseract.exe'
for i in range(rows):
for j in range(cols):
img_path = f'cell_{i}_{j}.jpg'
img = cv2.imread(img_path)
text = pytesseract.image_to_string(img)
print(f'[{i}, {j}]: {text}')
```
需要注意的是,该代码示例仅适用于简单的表格,对于复杂的表格可能需要更加复杂的算法和代码来处理。同时,OCR识别的准确率也可能会受到图像质量、字体、字号等因素的影响。
阅读全文
相关推荐
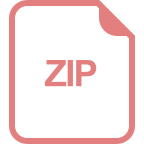
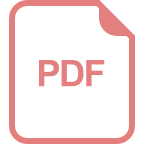
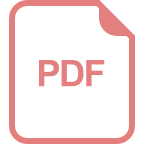
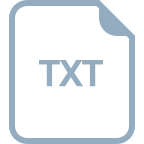
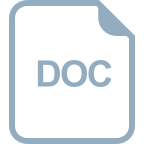
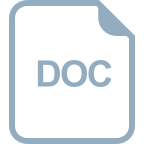
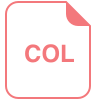
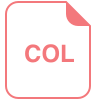
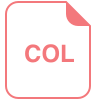
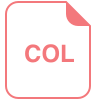
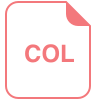
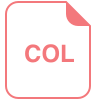
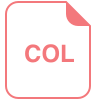
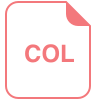
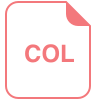
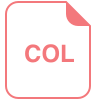
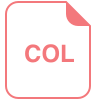
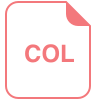