能给我写一段能实现该功能的代码吗
时间: 2024-01-08 14:02:57 浏览: 25
以下是一个基于C++和pugixml库的简单实现示例:
```c++
#include <iostream>
#include <string>
#include "pugixml.hpp"
using namespace std;
using namespace pugi;
// 定义数据结构
struct TLINK {
string id;
string event1_id;
string event2_id;
string relation_type;
};
// 读取XML文件并解析标注结果
void readTLINK(string filename, vector<TLINK>& tlinks) {
// 加载XML文件
xml_document doc;
if (!doc.load_file(filename.c_str())) {
cerr << "Error loading XML file " << filename << endl;
return;
}
// 获取root节点
xml_node root = doc.child("TimeML");
// 遍历TLINK节点
for (xml_node tlink = root.child("TLINK"); tlink; tlink = tlink.next_sibling("TLINK")) {
TLINK tl;
tl.id = tlink.attribute("id").value();
tl.event1_id = tlink.attribute("event1").value();
tl.event2_id = tlink.attribute("event2").value();
tl.relation_type = tlink.attribute("relType").value();
tlinks.push_back(tl);
}
}
// 应用标注结果到原始文本
void applyTLINK(string text, vector<TLINK>& tlinks) {
// TODO: 实现应用标注结果到原始文本的代码
}
int main() {
string filename = "tlink.xml";
vector<TLINK> tlinks;
readTLINK(filename, tlinks);
// TODO: 获取原始文本并将标注结果应用到原始文本中
return 0;
}
```
在该代码中,readTLINK函数用于读取XML文件并解析标注结果,将解析结果存储在TLINK结构体的vector中。applyTLINK函数用于将标注结果应用到原始文本中,这里暂时未实现。
在主函数中,我们首先指定标注结果的XML文件名,然后调用readTLINK函数读取标注结果。接下来,我们需要获取原始文本并将标注结果应用到原始文本中,这里需要根据具体的项目进行具体实现。
相关推荐
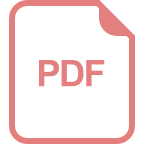
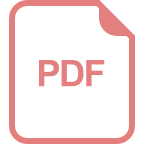














