用css 画一排小圆点,每两个小圆点间间隔10px
时间: 2024-03-09 10:29:01 浏览: 140
可以使用伪元素 `::before` 和 `content` 属性来实现:
HTML:
```html
<div class="circle-row"></div>
```
CSS:
```css
.circle-row {
display: flex;
}
.circle-row::before {
content: "";
flex: 1;
}
.circle-row span {
width: 10px;
height: 10px;
border-radius: 50%;
background-color: black;
margin: 0 5px;
}
```
解释:
- `display: flex;` 将 `.circle-row` 容器设置为弹性布局。
- `::before` 伪元素可以在 `.circle-row` 容器前插入一个空元素。
- `content: "";` 在伪元素中添加空内容。
- `flex: 1;` 将伪元素设置为占满剩余空间。
- `width: 10px; height: 10px; border-radius: 50%; background-color: black;` 将圆点设置为宽高为 10px、圆角为 50%、背景为黑色的圆形。
- `margin: 0 5px;` 将圆点之间的间距设为 10px(两个圆点间需要 5px 的 margin)。
效果如下:

相关问题
react 画一个小圆点
在React中,你可以使用内置的HTML元素`<svg>`或者第三方库如`react-icons`来绘制小圆点。这里是一个简单的例子,我们将使用SVG:
```jsx
import React from 'react';
function SmallDot() {
return (
<svg xmlns="http://www.w3.org/2000/svg" width="16" height="16">
<circle cx="8" cy="8" r="4" fill="#FF0000" />
</svg>
);
}
export default SmallDot;
```
这个组件会渲染一个红色的小圆点,其中心坐标为(8, 8),半径为4。如果你想让它更具交互性,可以添加事件监听。
如果你想要更现代一些,也可以考虑使用CSS样式来自定义圆点:
```jsx
import React from 'react';
import './SmallDot.css'; // 假设在另一个文件中有`.dot { ... }`的样式
function SmallDot() {
return <div className="dot" />;
}
export default SmallDot;
```
然后在`.dot` CSS类中定义圆点样式:
```css
.dot {
width: 20px;
height: 20px;
border-radius: 50%;
background-color: red;
}
```
写一个css动态散光小圆点
好的,我可以为您提供一个实现动态散光小圆点效果的CSS代码:
HTML代码:
```
<div class="container">
<div class="circle"></div>
</div>
```
CSS代码:
```
.container {
position: relative;
width: 300px;
height: 300px;
}
.circle {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 10px;
height: 10px;
border-radius: 50%;
background: #fff;
box-shadow: 0 0 5px 2px rgba(255,255,255,0.7), 0 0 10px 5px rgba(255,255,255,0.5), 0 0 20px 10px rgba(255,255,255,0.2), 0 0 40px 20px rgba(255,255,255,0.1), 0 0 80px 40px rgba(255,255,255,0.05);
animation: pulse 2s ease-out infinite;
}
@keyframes pulse {
0% {
width: 10px;
height: 10px;
opacity: 1;
}
50% {
width: 20px;
height: 20px;
opacity: 0.5;
}
100% {
width: 10px;
height: 10px;
opacity: 1;
}
}
```
这段CSS代码实现了一个圆形的小圆点,通过box-shadow的阴影效果,实现了动态散光的效果。同时通过animation实现了动态缩放的效果。您可以将container的宽高调整为适合您需要的大小。
阅读全文
相关推荐
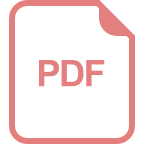
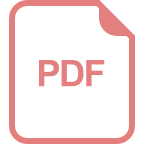












