python threading local
时间: 2023-08-24 16:07:10 浏览: 44
In Python, the `threading.local()` class creates an object that can store data that is local to a specific thread. This is useful when you have shared resources that need to be accessed by multiple threads, but each thread should have its own copy of the resource.
To use `threading.local()`, you first create an instance of the `threading.local()` class. Then, you can access and set attributes on the instance, just like you would with any other object. However, each thread will have its own instance of the object, so changes made in one thread will not affect the object in other threads.
Here's an example:
```python
import threading
# create a local object
local_data = threading.local()
# set a value in the local object
local_data.value = 42
def some_function():
# access the local object and print its value
print(local_data.value)
# create two threads that call the function
thread1 = threading.Thread(target=some_function)
thread2 = threading.Thread(target=some_function)
# start the threads
thread1.start()
thread2.start()
# wait for the threads to finish
thread1.join()
thread2.join()
```
In this example, we create a local object called `local_data` using the `threading.local()` class. We then set a value called `value` on the local object.
We define a function called `some_function()` which simply prints the value of `local_data.value`. We then create two threads that call this function.
When we start the threads, each thread will have its own instance of `local_data`, with its own copy of the `value` attribute set to 42. When the threads call `some_function()`, they will print their own copy of the `value` attribute, which will always be 42 in this case.
By using `threading.local()`, we can ensure that each thread has its own copy of the shared resource, preventing race conditions and other concurrency issues.
相关推荐
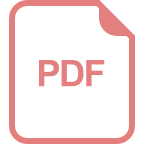
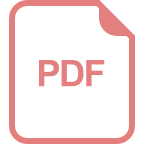
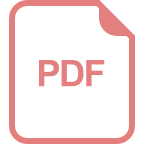















