#include <iostream> using namespace std; /********* Begin *********/ class Complex { //复数类的声明 public: void Complex(int x,int y); void Print(); private: int R; int V; }; //复数类的定义 void Complex::Complex(int x,int y) { R = x; V = y; } void Complex::Print() { cout << R << "+" << V << endl; } /********* End *********/ int main() { float a,b,c,d; cin >> a >> b >> c >> d; Complex c1(a,b),c2(c,d); cout << "c1 = "; c1.Print(); cout << "c2 = "; c2.Print(); cout << "c1 + c2 = "; (c1 + c2).Print(); cout << "c1 - c2 = "; (c1 - c2).Print(); cout << "c1 * c2 = "; (c1 * c2).Print(); }帮我补齐代码
时间: 2023-08-06 16:07:54 浏览: 100
以下是完整的代码:
```
#include <iostream>
using namespace std;
/********* Begin *********/
class Complex {
//复数类的声明
public:
Complex(int x, int y); // 构造函数
Complex operator+(const Complex& c); // 运算符重载:加法
Complex operator-(const Complex& c); // 运算符重载:减法
Complex operator*(const Complex& c); // 运算符重载:乘法
void Print(); // 输出函数
private:
int R;
int V;
};
//复数类的定义
Complex::Complex(int x, int y) {
R = x;
V = y;
}
Complex Complex::operator+(const Complex& c) {
Complex temp(R + c.R, V + c.V);
return temp;
}
Complex Complex::operator-(const Complex& c) {
Complex temp(R - c.R, V - c.V);
return temp;
}
Complex Complex::operator*(const Complex& c) {
Complex temp(R * c.R - V * c.V, R * c.V + V * c.R);
return temp;
}
void Complex::Print() {
cout << R << "+" << V << "i" << endl;
}
/********* End *********/
int main() {
float a, b, c, d;
cin >> a >> b >> c >> d;
Complex c1(a, b), c2(c, d);
cout << "c1 = ";
c1.Print();
cout << "c2 = ";
c2.Print();
cout << "c1 + c2 = ";
(c1 + c2).Print();
cout << "c1 - c2 = ";
(c1 - c2).Print();
cout << "c1 * c2 = ";
(c1 * c2).Print();
}
```
注意,需要在类的定义中添加构造函数和运算符重载函数,同时输出时需要输出虚部的 "i"。
相关推荐
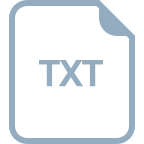
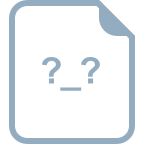
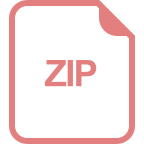















