怎样用c语言查看双链表的长度
时间: 2024-01-10 18:04:16 浏览: 24
要查看双链表的长度,需要遍历整个链表并计算节点数。下面是一个示例代码,使用了一个计数器变量来统计节点数:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* prev;
struct Node* next;
};
int getLength(struct Node* head) {
int count = 0;
struct Node* current = head;
while (current != NULL) {
count++;
current = current->next;
}
return count;
}
int main() {
// 创建一个双链表
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
head = (struct Node*)malloc(sizeof(struct Node));
second = (struct Node*)malloc(sizeof(struct Node));
third = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->prev = NULL;
head->next = second;
second->data = 2;
second->prev = head;
second->next = third;
third->data = 3;
third->prev = second;
third->next = NULL;
// 获取链表长度并输出
int length = getLength(head);
printf("Length of the linked list is: %d\n", length);
// 释放内存
free(head);
free(second);
free(third);
return 0;
}
```
以上代码创建了一个包含三个节点的双链表,并使用 `getLength()` 函数来获取链表的长度,并输出结果。
相关推荐
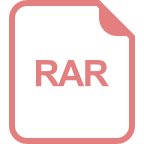
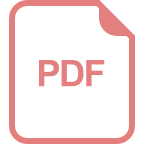
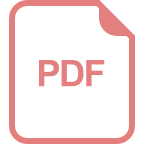















