1.定义一个单词库,可以使用数组或者文件读取的方式来实现。使用随机数来从单词库中随机选择一个单词作为答案。 2.定义一个函数来生成需要猜测的单词或组合。可以使用字符串或者字符数组来表示答案和猜测的单词或组合。对于答案中的每个字母,将其替换成“”表示未猜出来。如果答案中有重复的字母,需要全部替换成“”。 3.定义一个函数来判断玩家或电脑猜测的字母或组合是否在答案中出现。对于每次猜测,需要遍历答案中的所有字母,判断是否与猜测的字母或组合相同。如果相同,需要更新猜测的单词或组合情况,并显示正确的字母或组合。如果不同,需要显示已经猜错的字母,并减少剩余猜错次数。当剩余猜错次数为 0 时,游戏结束,显示正确答案并询问是否重新开始游戏。如果猜测正确,则游戏胜利,显示胜利信息,并询问是否重新开始游戏。 4.使用一个循环来实现是否重新开始游戏的功能。当游戏结束时,询问玩家是否继续游戏,如果选择是,则重新开始游戏。否则退出程序。c++
时间: 2023-12-10 21:42:28 浏览: 63
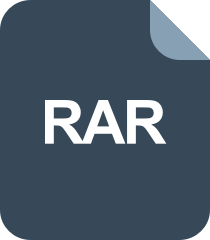
菜鸟也可以实现的“背单词”程序.rar
以下是一个简单的 C++ 猜单词游戏的代码示例:
```c++
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
const string WORDS[] = {"apple", "banana", "orange", "peach", "watermelon", "grape", "kiwi", "pineapple", "strawberry", "pear"};
const int WORDS_LENGTH = sizeof(WORDS) / sizeof(string);
string selectWord()
{
srand(time(NULL));
int index = rand() % WORDS_LENGTH;
return WORDS[index];
}
string hideWord(string word)
{
string hidden = "";
for (int i = 0; i < word.length(); i++)
{
if (word.find(word[i]) == i)
{
hidden += "_";
}
else
{
hidden += "";
}
}
return hidden;
}
bool isGuessCorrect(string word, string guess, char letter)
{
bool correct = false;
for (int i = 0; i < word.length(); i++)
{
if (word[i] == letter)
{
guess[i] = letter;
correct = true;
}
}
return correct;
}
int main()
{
string word = selectWord();
string hidden = hideWord(word);
int remainingGuesses = 6;
bool gameWon = false;
cout << "Welcome to the Guess the Word Game!" << endl;
cout << "The word has " << word.length() << " letters, and you have " << remainingGuesses << " guesses remaining." << endl;
while (remainingGuesses > 0)
{
cout << "Guess a letter: ";
char letter;
cin >> letter;
if (isGuessCorrect(word, hidden, letter))
{
cout << "Correct: " << hidden << endl;
if (hidden == word)
{
gameWon = true;
break;
}
}
else
{
remainingGuesses--;
cout << "Incorrect, " << remainingGuesses << " guesses remaining." << endl;
}
}
if (gameWon)
{
cout << "Congratulations, you guessed the word!" << endl;
}
else
{
cout << "Sorry, you ran out of guesses. The word was " << word << "." << endl;
}
cout << "Would you like to play again? (y/n): ";
char playAgain;
cin >> playAgain;
if (playAgain == 'y')
{
main();
}
return 0;
}
```
在该代码中,我们首先定义了一个单词库 `WORDS` 和其长度 `WORDS_LENGTH`。然后,我们使用 `selectWord` 函数随机选择一个单词作为答案。接着,我们使用 `hideWord` 函数将答案中的每个字母替换成“_”表示未猜出来。`isGuessCorrect` 函数用于判断玩家或电脑猜测的字母或组合是否在答案中出现,并更新猜测的单词或组合情况。最后,我们使用一个循环来实现游戏的主要逻辑,并询问玩家是否继续游戏。
阅读全文
相关推荐
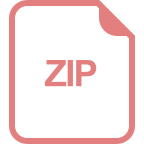
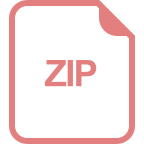

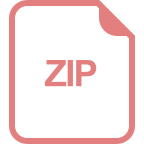
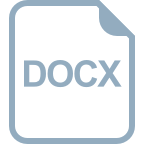
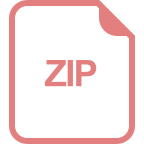
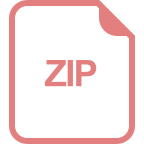
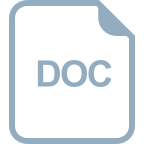
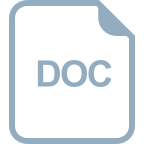
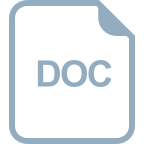
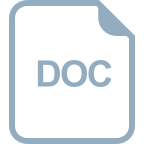
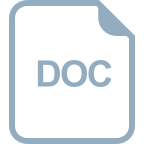
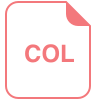
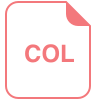



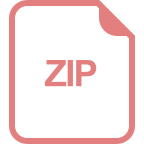