用c语言解决:现有3本图书,每本图书的信息包括:书号、书名、作者、价格。 (1)编写input函数,实现从键盘输入若干本图书信息,存放在结构体数组中。 (2)编写output函数,遍历结构体数组,输出所有图书信息。 (3)编写findBookByID函数,实现按书号检索图书。 (4)编写findBookByName函数,实现按书名检索图书。
时间: 2024-02-11 20:06:47 浏览: 61
好的,您的问题我已经记下了。以下是使用C语言编写的解决方案:
```c
#include <stdio.h>
#include <string.h>
#define MAX_BOOKS 3
#define MAX_NAME_LEN 50
#define MAX_AUTHOR_LEN 30
struct Book {
int id;
char name[MAX_NAME_LEN];
char author[MAX_AUTHOR_LEN];
float price;
};
struct Book books[MAX_BOOKS];
int count = 0;
// 从键盘输入若干本图书信息,存放在结构体数组中
void input() {
for (int i = 0; i < MAX_BOOKS; i++) {
printf("请输入第%d本书的信息:\n", i+1);
printf("书号:");
scanf("%d", &books[i].id);
printf("书名:");
scanf("%s", books[i].name);
printf("作者:");
scanf("%s", books[i].author);
printf("价格:");
scanf("%f", &books[i].price);
count++;
}
}
// 遍历结构体数组,输出所有图书信息
void output() {
printf("所有图书信息如下:\n");
for (int i = 0; i < count; i++) {
printf("书号:%d\n", books[i].id);
printf("书名:%s\n", books[i].name);
printf("作者:%s\n", books[i].author);
printf("价格:%.2f\n", books[i].price);
printf("\n");
}
}
// 按书号检索图书
void findBookByID(int id) {
for (int i = 0; i < count; i++) {
if (books[i].id == id) {
printf("书号:%d\n", books[i].id);
printf("书名:%s\n", books[i].name);
printf("作者:%s\n", books[i].author);
printf("价格:%.2f\n", books[i].price);
return;
}
}
printf("没有找到书号为%d的图书\n", id);
}
// 按书名检索图书
void findBookByName(char* name) {
for (int i = 0; i < count; i++) {
if (strcmp(books[i].name, name) == 0) {
printf("书号:%d\n", books[i].id);
printf("书名:%s\n", books[i].name);
printf("作者:%s\n", books[i].author);
printf("价格:%.2f\n", books[i].price);
return;
}
}
printf("没有找到书名为%s的图书\n", name);
}
int main() {
input();
output();
findBookByID(2);
findBookByName("C语言程序设计");
return 0;
}
```
以上代码中,我们先定义了一个结构体`Book`,用于存放每本图书的信息。然后定义了一个结构体数组`books`,用于存放所有图书的信息。`count`变量用于记录当前已经输入了多少本图书。
然后编写了`input`函数,可以从键盘输入若干本图书信息,并将其存放在`books`数组中。
接着编写了`output`函数,用于遍历`books`数组,输出所有图书的信息。
然后编写了`findBookByID`函数和`findBookByName`函数,分别实现按书号和按书名检索图书的功能。
最后在`main`函数中,我们调用了以上编写的函数,来实现对图书信息的输入、输出、检索等操作。
相关推荐
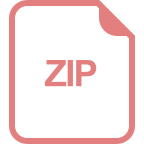
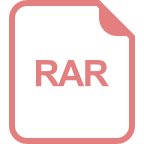














