现有3本图书,每本图书的信息包括:书号、书名、作者、价格。 (1)编写input函数,实现从键盘输入若干本图书信息,存放在结构体数组中。 (2)编写output函数,遍历结构体数组,输出所有图书信息。 (3)编写findBookByID函数,实现按书号检索图书。 (4)编写findBookByName函数,实现按书名检索图书。 c语言
时间: 2023-12-10 17:36:55 浏览: 70
以下是实现上述功能的C语言代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_BOOKS 100
struct Book {
char id[20];
char name[50];
char author[50];
float price;
};
struct Book books[MAX_BOOKS];
int num_books = 0;
void input() {
while (num_books < MAX_BOOKS) {
printf("Enter book info (id name author price): ");
scanf("%s %s %s %f", books[num_books].id, books[num_books].name, books[num_books].author, &books[num_books].price);
num_books++;
char c;
printf("Do you want to continue (y/n)? ");
scanf(" %c", &c);
if (c == 'n' || c == 'N') {
break;
}
}
}
void output() {
printf("Book list:\n");
for (int i = 0; i < num_books; i++) {
printf("%s %s %s %.2f\n", books[i].id, books[i].name, books[i].author, books[i].price);
}
}
void findBookByID(char id[]) {
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].id, id) == 0) {
printf("%s %s %s %.2f\n", books[i].id, books[i].name, books[i].author, books[i].price);
return;
}
}
printf("Book not found\n");
}
void findBookByName(char name[]) {
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].name, name) == 0) {
printf("%s %s %s %.2f\n", books[i].id, books[i].name, books[i].author, books[i].price);
}
}
}
int main() {
input();
output();
char id[20];
printf("Enter book id to search: ");
scanf("%s", id);
findBookByID(id);
char name[50];
printf("Enter book name to search: ");
scanf("%s", name);
findBookByName(name);
return 0;
}
```
这里我们定义了一个结构体类型 `struct Book`,用于表示图书的信息。在 `input()` 函数中,我们使用循环从键盘输入图书信息,并将它们存储在 `books` 数组中。在 `output()` 函数中,我们遍历 `books` 数组,输出所有图书信息。在 `findBookByID()` 和 `findBookByName()` 函数中,我们分别按书号和书名检索图书,并输出符合条件的图书信息。在 `main()` 函数中,我们调用上述函数来完成对图书信息的输入、输出和检索。
相关推荐
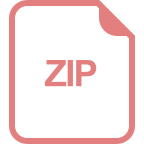
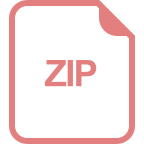














