c语言代码函数名称:findBookByID * 函数功能:按书号检索图书 * 形式参数:p,book型指针,book型一维数组首地址 * 形式参数:num,int型,图书数量 * 形式参数:id,int型,书号 * 返 回 值:返回book型指针。若没有找到图书,则返回空指针NULL;否则,仅返回第1本符合检索条件的图书 */ struct book * findBookByID(struct book *p, int num, int id){ // 请编程实现本函数#include <stdio.h> #include <string.h> typedef struct book{ int id; // 书号 char name[30]; // 书名 char author[30]; //作者 float price; //价格 }BOOK; int main(){ /* 声明函数 */ void input(struct book *p, int num); void output(struct book *p, int num); struct book * findBookByID(struct book *p, int num, int id); struct book * findBookByName(struct book p, int num, char name[]); / 声明变量 */ const int NUM = 3; struct book myBooks[NUM]; struct book *pBook = myBooks; BOOK theBook; / 为myBooks数组的所有元素赋值 / input(pBook, NUM); / 按ID检索图书 / int bookID; scanf("%d", &bookID); fflush(stdin); /清空输入缓冲区/ theBook = findBookByID(pBook, NUM, bookID); /按书号检索/ output(theBook, 1); /输出检索结果/ / 输出myBooks数组的所有元素值 / output(pBook, NUM); return 0; } / *
时间: 2024-02-06 11:02:22 浏览: 122
根据您提供的代码,以下是完整的 C 语言程序:
```
#include <stdio.h>
#include <string.h>
typedef struct book{
int id; // 书号
char name[30]; // 书名
char author[30]; // 作者
float price; // 价格
}BOOK;
/* 按书号检索图书 */
struct book *findBookByID(struct book *p, int num, int id){
for (int i = 0; i < num; i++) {
if (p[i].id == id) {
return &p[i]; // 返回第1本符合检索条件的图书
}
}
return NULL; // 没有找到图书,返回空指针
}
/* 声明函数 */
void input(struct book *p, int num);
void output(struct book *p, int num);
struct book *findBookByName(struct book p, int num, char name[]);
int main(){
const int NUM = 3;
struct book myBooks[NUM];
struct book *pBook = myBooks;
BOOK theBook;
/* 为 myBooks 数组的所有元素赋值 */
input(pBook, NUM);
/* 按 ID 检索图书 */
int bookID;
scanf("%d", &bookID);
fflush(stdin); // 清空输入缓冲区
theBook = *findBookByID(pBook, NUM, bookID); // 按书号检索
output(&theBook, 1); // 输出检索结果
/* 输出 myBooks 数组的所有元素值 */
output(pBook, NUM);
return 0;
}
/* 从键盘输入图书信息 */
void input(struct book *p, int num){
for (int i = 0; i < num; i++) {
printf("请输入第 %d 本书信息:\n", i + 1);
printf("书号:");
scanf("%d", &p[i].id);
fflush(stdin); // 清空输入缓冲区
printf("书名:");
gets(p[i].name);
printf("作者:");
gets(p[i].author);
printf("价格:");
scanf("%f", &p[i].price);
fflush(stdin); // 清空输入缓冲区
}
}
/* 输出图书信息 */
void output(struct book *p, int num){
printf("图书信息如下:\n");
for (int i = 0; i < num; i++) {
printf("书号:%d,书名:%s,作者:%s,价格:%.2f\n", p[i].id, p[i].name, p[i].author, p[i].price);
}
}
/* 按书名检索图书 */
struct book *findBookByName(struct book *p, int num, char name[]){
for (int i = 0; i < num; i++) {
if (strcmp(p[i].name, name) == 0) {
return &p[i]; // 返回第1本符合检索条件的图书
}
}
return NULL; // 没有找到图书,返回空指针
}
```
该程序实现了按书号检索图书的功能,同时还包含了按书名检索图书的函数 `findBookByName`,以及从键盘输入图书信息和输出图书信息的函数 `input` 和 `output`。在 `main` 函数中,用户输入要检索的图书的书号,然后调用 `findBookByID` 函数查找图书并输出检索结果,最后输出所有图书的信息。
阅读全文
相关推荐
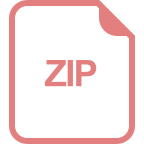



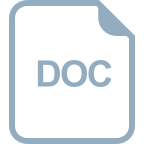
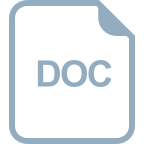
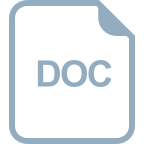
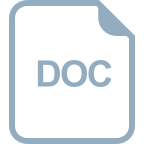
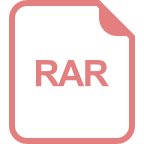
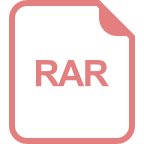
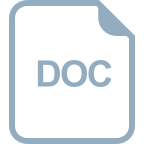
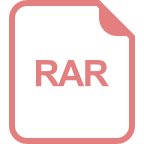
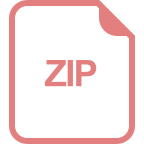
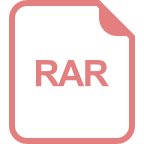
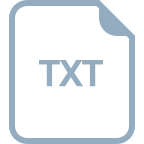