c语言代码:#include <stdio.h> #include <string.h> typedef struct book{ int id; // 书号 char name[30]; // 书名 char author[30]; //作者 float price; //价格 }BOOK; int main(){ /* 声明函数 */ void input(struct book *p, int num); void output(struct book *p, int num); struct book * findBookByID(struct book *p, int num, int id); struct book * findBookByName(struct book *p, int num, char name[]); /* 声明变量 */ const int NUM = 3; struct book myBooks[NUM]; struct book *pBook = myBooks; BOOK *theBook; /* 为myBooks数组的所有元素赋值 */ input(pBook, NUM); /* 按ID检索图书 */ int bookID; scanf("%d", &bookID); fflush(stdin); /*清空输入缓冲区*/ theBook = findBookByID(pBook, NUM, bookID); /*按书号检索*/ output(theBook, 1); /*输出检索结果*/ /* 输出myBooks数组的所有元素值 */ output(pBook, NUM); return 0;* * 函数名称:findBookByID * 函数功能:按书号检索图书 * 形式参数:p,book型指针,book型一维数组首地址 * 形式参数:num,int型,图书数量 * 形式参数:id,int型,书号 * 返 回 值:返回book型指针。若没有找到图书,则返回空指针NULL;否则,仅返回第1本符合检索条件的图书 */ struct book * findBookByID(struct book *p, int num, int id){ // 请编程实现本函数
时间: 2024-02-04 07:02:21 浏览: 21
首先我们需要遍历整个书的数组,查找是否有书号与要查找的书号相同的书。如果找到了符合要求的书,就返回该书的指针;否则返回空指针NULL。
具体实现:
```
struct book *findBookByID(struct book *p, int num, int id){
for(int i = 0; i < num; i++){
if(p[i].id == id){
return &p[i];
}
}
return NULL;
}
```
相关问题
c语言代码函数名称:findBookByID * 函数功能:按书号检索图书 * 形式参数:p,book型指针,book型一维数组首地址 * 形式参数:num,int型,图书数量 * 形式参数:id,int型,书号 * 返 回 值:返回book型指针。若没有找到图书,则返回空指针NULL;否则,仅返回第1本符合检索条件的图书 */ struct book * findBookByID(struct book *p, int num, int id){ // 请编程实现本函数#include <stdio.h> #include <string.h> typedef struct book{ int id; // 书号 char name[30]; // 书名 char author[30]; //作者 float price; //价格 }BOOK; int main(){ /* 声明函数 */ void input(struct book *p, int num); void output(struct book *p, int num); struct book * findBookByID(struct book *p, int num, int id); struct book * findBookByName(struct book p, int num, char name[]); / 声明变量 */ const int NUM = 3; struct book myBooks[NUM]; struct book *pBook = myBooks; BOOK theBook; / 为myBooks数组的所有元素赋值 / input(pBook, NUM); / 按ID检索图书 / int bookID; scanf("%d", &bookID); fflush(stdin); /清空输入缓冲区/ theBook = findBookByID(pBook, NUM, bookID); /按书号检索/ output(theBook, 1); /输出检索结果/ / 输出myBooks数组的所有元素值 / output(pBook, NUM); return 0; } / *
根据您提供的代码,以下是完整的 C 语言程序:
```
#include <stdio.h>
#include <string.h>
typedef struct book{
int id; // 书号
char name[30]; // 书名
char author[30]; // 作者
float price; // 价格
}BOOK;
/* 按书号检索图书 */
struct book *findBookByID(struct book *p, int num, int id){
for (int i = 0; i < num; i++) {
if (p[i].id == id) {
return &p[i]; // 返回第1本符合检索条件的图书
}
}
return NULL; // 没有找到图书,返回空指针
}
/* 声明函数 */
void input(struct book *p, int num);
void output(struct book *p, int num);
struct book *findBookByName(struct book p, int num, char name[]);
int main(){
const int NUM = 3;
struct book myBooks[NUM];
struct book *pBook = myBooks;
BOOK theBook;
/* 为 myBooks 数组的所有元素赋值 */
input(pBook, NUM);
/* 按 ID 检索图书 */
int bookID;
scanf("%d", &bookID);
fflush(stdin); // 清空输入缓冲区
theBook = *findBookByID(pBook, NUM, bookID); // 按书号检索
output(&theBook, 1); // 输出检索结果
/* 输出 myBooks 数组的所有元素值 */
output(pBook, NUM);
return 0;
}
/* 从键盘输入图书信息 */
void input(struct book *p, int num){
for (int i = 0; i < num; i++) {
printf("请输入第 %d 本书信息:\n", i + 1);
printf("书号:");
scanf("%d", &p[i].id);
fflush(stdin); // 清空输入缓冲区
printf("书名:");
gets(p[i].name);
printf("作者:");
gets(p[i].author);
printf("价格:");
scanf("%f", &p[i].price);
fflush(stdin); // 清空输入缓冲区
}
}
/* 输出图书信息 */
void output(struct book *p, int num){
printf("图书信息如下:\n");
for (int i = 0; i < num; i++) {
printf("书号:%d,书名:%s,作者:%s,价格:%.2f\n", p[i].id, p[i].name, p[i].author, p[i].price);
}
}
/* 按书名检索图书 */
struct book *findBookByName(struct book *p, int num, char name[]){
for (int i = 0; i < num; i++) {
if (strcmp(p[i].name, name) == 0) {
return &p[i]; // 返回第1本符合检索条件的图书
}
}
return NULL; // 没有找到图书,返回空指针
}
```
该程序实现了按书号检索图书的功能,同时还包含了按书名检索图书的函数 `findBookByName`,以及从键盘输入图书信息和输出图书信息的函数 `input` 和 `output`。在 `main` 函数中,用户输入要检索的图书的书号,然后调用 `findBookByID` 函数查找图书并输出检索结果,最后输出所有图书的信息。
基于链式存储结构的图书信息表的最爱图书的查找C语言代码
以下是基于链式存储结构的图书信息表的最爱图书的查找C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义图书信息结构体
typedef struct Book {
char id[10]; // 书号
char name[50]; // 书名
float price; // 价格
struct Book *next; // 指向下一个节点的指针
} Book;
// 创建图书信息链表
Book *createBookList(int n) {
Book *head, *p, *q;
head = (Book *)malloc(sizeof(Book));
head->next = NULL;
q = head;
for (int i = 0; i < n; i++) {
p = (Book *)malloc(sizeof(Book));
printf("请输入第%d本书的信息:\n", i + 1);
printf("书号:");
scanf("%s", p->id);
printf("书名:");
scanf("%s", p->name);
printf("价格:");
scanf("%f", &p->price);
q->next = p;
q = p;
}
q->next = NULL;
return head;
}
// 查找最爱的图书
Book *findFavoriteBook(Book *head, char *favoriteBookName) {
Book *p = head->next;
while (p != NULL) {
if (strcmp(p->name, favoriteBookName) == 0) {
return p;
}
p = p->next;
}
return NULL;
}
// 输出图书信息
void printBookInfo(Book *book) {
printf("书号:%s\n", book->id);
printf("书名:%s\n", book->name);
printf("价格:%.2f\n", book->price);
}
int main() {
int n;
char favoriteBookName[50];
Book *head, *favoriteBook;
printf("请输入图书数量:");
scanf("%d", &n);
head = createBookList(n);
printf("请输入最爱的图书名字:");
scanf("%s", favoriteBookName);
favoriteBook = findFavoriteBook(head, favoriteBookName);
if (favoriteBook != NULL) {
printf("您最爱的图书信息如下:\n");
printBookInfo(favoriteBook);
} else {
printf("抱歉,没有找到您最爱的图书。\n");
}
return 0;
}
```
相关推荐
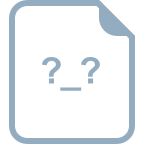
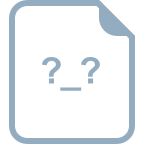












