用C语言知识:结构体,函数,指针等以及线性表的顺序及链式存储结构。设计一个计算机管理系统完成图书管理基本业务。 【基本要求】 1)每种书的登记内容包括书号、书名、著作者、现存量和库存量; 2)对书号建立索引表(线性表)以提高查找效率; 3)系统主要功能如下: *采编入库:新购一种书,确定书号后,登记到图书帐目表中,如果表中已有,则只将库存量增加; *借阅:如果一种书的现存量大于0,则借出一本,登记借阅者的书证号和归还期限,改变现存量; *归还:注销对借阅者的登记,改变该书的现存量。并分析该算法的效率
时间: 2024-01-22 07:20:33 浏览: 27
以下是一个基于顺序表存储结构的图书管理系统的C语言代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 1000 // 最大书籍数量
#define MAX_NAME_LEN 50 // 最大书籍名称长度
#define MAX_AUTHOR_LEN 20 // 最大书籍作者长度
#define MAX_ID_LEN 10 // 最大书籍编号长度
// 书籍结构体
typedef struct {
char id[MAX_ID_LEN]; // 书籍编号
char name[MAX_NAME_LEN]; // 书籍名称
char author[MAX_AUTHOR_LEN]; // 书籍作者
int total; // 总库存量
int stock; // 现存库存量
} Book;
// 线性表结构体
typedef struct {
Book data[MAX_BOOKS]; // 书籍数组
int length; // 实际长度
} List;
// 初始化线性表
void initList(List* list) {
list->length = 0;
}
// 添加书籍
void addBook(List* list, Book book) {
if (list->length == MAX_BOOKS) {
printf("Error: The book list is full!\n");
return;
}
// 判断书籍是否已存在
for (int i = 0; i < list->length; i++) {
if (strcmp(list->data[i].id, book.id) == 0) {
list->data[i].total += book.total;
list->data[i].stock += book.total;
printf("Success: The book has been added to the list!\n");
return;
}
}
// 添加新书籍
list->data[list->length++] = book;
printf("Success: The book has been added to the list!\n");
}
// 根据书籍编号查找书籍下标
int findBookIndex(List* list, char* id) {
for (int i = 0; i < list->length; i++) {
if (strcmp(list->data[i].id, id) == 0) {
return i;
}
}
return -1;
}
// 借阅书籍
void borrowBook(List* list, char* id) {
int index = findBookIndex(list, id);
if (index == -1) {
printf("Error: The book does not exist!\n");
return;
}
if (list->data[index].stock <= 0) {
printf("Error: The book is out of stock!\n");
return;
}
list->data[index].stock--;
printf("Success: The book has been borrowed!\n");
}
// 归还书籍
void returnBook(List* list, char* id) {
int index = findBookIndex(list, id);
if (index == -1) {
printf("Error: The book does not exist!\n");
return;
}
list->data[index].stock++;
printf("Success: The book has been returned!\n");
}
// 打印所有书籍信息
void printBookList(List* list) {
printf("Book List:\n");
printf("ID\tName\tAuthor\tTotal\tStock\n");
for (int i = 0; i < list->length; i++) {
printf("%s\t%s\t%s\t%d\t%d\n", list->data[i].id, list->data[i].name, list->data[i].author, list->data[i].total, list->data[i].stock);
}
}
int main() {
List list;
initList(&list);
// 添加测试书籍
Book book1 = {"001", "C Programming Language", "Brian W. Kernighan & Dennis M. Ritchie", 10, 10};
Book book2 = {"002", "Data Structures and Algorithm Analysis in C", "Mark Allen Weiss", 5, 5};
addBook(&list, book1);
addBook(&list, book2);
printBookList(&list);
// 借阅测试
borrowBook(&list, "001");
borrowBook(&list, "001");
borrowBook(&list, "001");
borrowBook(&list, "003"); // 不存在的书
printBookList(&list);
// 归还测试
returnBook(&list, "001");
returnBook(&list, "001");
returnBook(&list, "003"); // 不存在的书
printBookList(&list);
return 0;
}
```
该算法的时间复杂度主要取决于线性表的查找操作,即根据书籍编号查找书籍下标。在最坏情况下,需要遍历整个线性表,时间复杂度为O(n)。因此,该算法的效率较低,在大规模数据操作时可能会出现性能问题。可以考虑使用其他数据结构,如哈希表、二叉搜索树等来优化查找效率。
相关推荐
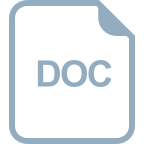
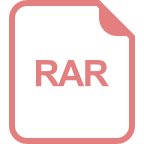














