用c写一个链式存储的线性表
时间: 2024-05-08 20:15:14 浏览: 16
以下是使用C语言实现链式存储的线性表的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data; // 数据域
struct ListNode *next; // 指针域
} ListNode;
// 定义链表结构体
typedef struct LinkedList {
ListNode *head; // 链表头节点指针
int length; // 链表长度
} LinkedList;
// 初始化链表
void initLinkedList(LinkedList *list) {
// 创建头节点
ListNode *head = (ListNode*)malloc(sizeof(ListNode));
head->data = 0;
head->next = NULL;
// 初始化链表
list->head = head;
list->length = 0;
}
// 插入元素
void insertElement(LinkedList *list, int data, int index) {
if (index < 0 || index > list->length) {
printf("Insert failed: Index out of bounds.\n");
return;
}
// 创建新节点
ListNode *node = (ListNode*)malloc(sizeof(ListNode));
node->data = data;
node->next = NULL;
// 找到插入位置的前一个节点
ListNode *prev = list->head;
for (int i = 0; i < index; i++) {
prev = prev->next;
}
// 插入新节点
node->next = prev->next;
prev->next = node;
// 更新链表长度
list->length++;
}
// 删除元素
void deleteElement(LinkedList *list, int index) {
if (index < 0 || index >= list->length) {
printf("Delete failed: Index out of bounds.\n");
return;
}
// 找到待删除节点的前一个节点
ListNode *prev = list->head;
for (int i = 0; i < index; i++) {
prev = prev->next;
}
// 删除节点
ListNode *node = prev->next;
prev->next = node->next;
free(node);
// 更新链表长度
list->length--;
}
// 获取元素
int getElement(LinkedList *list, int index) {
if (index < 0 || index >= list->length) {
printf("Get failed: Index out of bounds.\n");
return -1;
}
// 找到待获取节点
ListNode *node = list->head->next;
for (int i = 0; i < index; i++) {
node = node->next;
}
// 返回节点数据
return node->data;
}
// 打印链表
void printLinkedList(LinkedList *list) {
ListNode *node = list->head->next;
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
LinkedList list;
initLinkedList(&list);
insertElement(&list, 1, 0);
insertElement(&list, 2, 1);
insertElement(&list, 3, 2);
insertElement(&list, 4, 3);
printLinkedList(&list); // 输出:1 2 3 4
deleteElement(&list, 2);
printLinkedList(&list); // 输出:1 2 4
printf("Element at index 1: %d\n", getElement(&list, 1)); // 输出:2
return 0;
}
```
这个代码中,我们使用了两个结构体:`ListNode`和`LinkedList`。`ListNode`代表链表中的一个节点,包含一个数据域和一个指针域,指向下一个节点;`LinkedList`代表整个链表,包含一个头节点指针和链表长度。
在`initLinkedList`函数中,我们创建了头节点,并初始化了链表。
在`insertElement`函数中,我们根据传入的位置,找到插入位置的前一个节点,然后创建新节点,插入到链表中。
在`deleteElement`函数中,我们根据传入的位置,找到待删除节点的前一个节点,然后从链表中删除该节点。
在`getElement`函数中,我们根据传入的位置,找到待获取的节点,并返回该节点的数据域。
在`printLinkedList`函数中,我们遍历链表中的所有节点,并输出它们的数据域。
最后,在`main`函数中,我们演示了如何使用这些函数来操作链表。
相关推荐
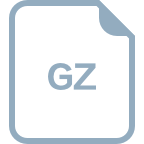
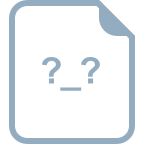














