用c语言写一段完整的链式存储的线性表的代码
时间: 2024-04-07 07:30:09 浏览: 118
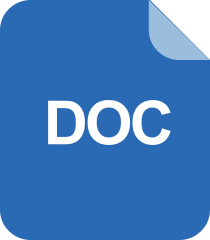
数据结构课程设计-成绩分析系统

当然!下面是一段使用C语言编写的链式存储的线性表代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct {
Node* head;
int length;
} LinkedList;
// 初始化链表
void initList(LinkedList* list) {
list->head = NULL;
list->length = 0;
}
// 判断链表是否为空
int isEmpty(LinkedList list) {
return list.head == NULL;
}
// 获取链表长度
int getLength(LinkedList list) {
return list.length;
}
// 在链表末尾插入元素
void insert(LinkedList* list, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (isEmpty(*list)) {
list->head = newNode;
} else {
Node* current = list->head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
list->length++;
}
// 删除链表指定位置的元素
int delete(LinkedList* list, int pos) {
if (isEmpty(*list) || pos < 1 || pos > list->length) {
return 0; // 删除失败
}
Node* temp;
if (pos == 1) {
temp = list->head;
list->head = list->head->next;
} else {
Node* current = list->head;
for (int i = 1; i < pos - 1; i++) {
current = current->next;
}
temp = current->next;
current->next = temp->next;
}
free(temp);
list->length--;
return 1; // 删除成功
}
// 获取链表指定位置的元素
int getElement(LinkedList list, int pos, int* value) {
if (isEmpty(list) || pos < 1 || pos > list.length) {
return 0; // 获取失败
}
Node* current = list.head;
for (int i = 1; i < pos; i++) {
current = current->next;
}
*value = current->data;
return 1; // 获取成功
}
// 输出链表的所有元素
void display(LinkedList list) {
if (isEmpty(list)) {
printf("链表为空\n");
return;
}
printf("链表元素为: ");
Node* current = list.head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
LinkedList list;
initList(&list);
insert(&list, 10);
insert(&list, 20);
insert(&list, 30);
display(list);
int value;
getElement(list, 2, &value);
printf("第2个位置的元素为: %d\n", value);
delete(&list, 2);
display(list);
return 0;
}
```
这段代码实现了链式存储的线性表的常用操作,包括初始化、判断是否为空、获取长度、在末尾插入元素、删除指定位置的元素、获取指定位置的元素和输出所有元素。在示例中,我们创建了一个链表,并插入了三个元素,然后输出链表的所有元素。接着获取第二个位置的元素,并删除第二个位置的元素,最后再次输出链表的所有元素。
阅读全文
相关推荐
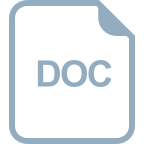
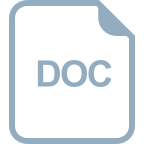






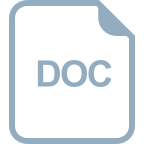








