用c++写一个整数单链表的建立、查找、插入、删除、输出程序(线性表的链式存储实现),表中不允许有重复数据,的程序
时间: 2023-10-19 07:12:36 浏览: 57
以下是一个简单的整数单链表的建立、查找、插入、删除、输出程序的示例,代码注释中有详细的说明:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data; // 数据域
struct ListNode *next; // 指针域
} ListNode;
// 创建链表
ListNode *createList() {
int n; // 链表中节点个数
ListNode *head, *tail; // 头节点和尾节点指针
// 创建头节点
head = (ListNode *)malloc(sizeof(ListNode));
head->next = NULL;
tail = head;
// 输入节点个数
printf("请输入链表中节点个数:");
scanf("%d", &n);
// 逐个插入节点
for (int i = 1; i <= n; i++) {
int x; // 当前节点的数据
ListNode *p = (ListNode *)malloc(sizeof(ListNode)); // 创建新节点
printf("请输入第%d个节点的数据:", i);
scanf("%d", &x);
p->data = x;
p->next = NULL;
tail->next = p; // 将新节点插入到尾节点后面
tail = p; // 更新尾节点指针
}
return head;
}
// 查找节点
ListNode *search(ListNode *head, int x) {
ListNode *p = head->next; // 指向第1个节点
while (p != NULL) {
if (p->data == x) {
return p; // 找到节点,返回指针
}
p = p->next; // 指向下一个节点
}
return NULL; // 没有找到节点,返回空指针
}
// 插入节点
void insert(ListNode *head, int x) {
ListNode *p = search(head, x); // 查找节点
if (p != NULL) {
printf("插入失败:链表中已经存在数据%d。\n", x);
return;
}
ListNode *newNode = (ListNode *)malloc(sizeof(ListNode)); // 创建新节点
newNode->data = x;
newNode->next = head->next; // 将新节点插入到头节点后面
head->next = newNode;
printf("插入成功:已在链表头插入数据%d。\n", x);
}
// 删除节点
void delete(ListNode *head, int x) {
ListNode *p = head->next; // 指向第1个节点
ListNode *prev = head; // p的前一个节点
while (p != NULL) {
if (p->data == x) {
prev->next = p->next; // 将p节点从链表中删除
free(p); // 释放p节点的内存
printf("删除成功:已从链表中删除数据%d。\n", x);
return;
}
prev = p; // prev指向p
p = p->next; // p指向下一个节点
}
printf("删除失败:链表中不存在数据%d。\n", x);
}
// 输出链表
void printList(ListNode *head) {
ListNode *p = head->next; // 指向第1个节点
printf("链表中的数据为:");
while (p != NULL) {
printf("%d ", p->data);
p = p->next; // 指向下一个节点
}
printf("\n");
}
int main() {
ListNode *head = createList(); // 创建链表
int x;
// 查找节点
printf("请输入要查找的数据:");
scanf("%d", &x);
ListNode *p = search(head, x);
if (p != NULL) {
printf("查找成功:在链表中找到数据%d。\n", x);
} else {
printf("查找失败:链表中没有数据%d。\n", x);
}
// 插入节点
printf("请输入要插入的数据:");
scanf("%d", &x);
insert(head, x);
printList(head);
// 删除节点
printf("请输入要删除的数据:");
scanf("%d", &x);
delete(head, x);
printList(head);
// 释放链表的内存
ListNode *p1 = head->next, *p2;
while (p1 != NULL) {
p2 = p1->next;
free(p1);
p1 = p2;
}
free(head);
return 0;
}
```
相关推荐
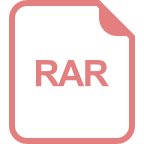
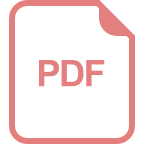
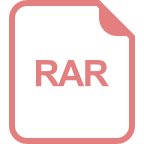














