2、整数单链表的建立、查找、插入、删除、输出程序(线性表的链式存储实现)
时间: 2023-09-28 12:10:14 浏览: 85
下面是整数单链表的建立、查找、插入、删除、输出程序(C++实现):
```cpp
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x): val(x), next(NULL) {}
};
// 建立链表函数
ListNode* createList() {
ListNode* head = new ListNode(0); // 创建头结点
ListNode* cur = head; // 创建当前结点指针,初始化指向头结点
int x;
while (cin >> x && x != -1) { // 输入-1表示结束
ListNode* newNode = new ListNode(x); // 创建新结点
cur->next = newNode; // 将新结点链接到当前结点后面
cur = cur->next; // 当前结点指针指向新结点
}
return head;
}
// 查找链表中是否存在某个元素
bool findElement(ListNode* head, int x) {
ListNode* cur = head->next; // 从第一个结点开始查找
while (cur != NULL) {
if (cur->val == x) {
return true; // 找到了
}
cur = cur->next; // 继续往后查找
}
return false; // 没找到
}
// 向链表中插入一个元素
void insertElement(ListNode* head, int pos, int x) {
ListNode* cur = head; // 从头结点开始查找
for (int i = 0; i < pos && cur != NULL; i++) { // 找到要插入位置的前一个结点
cur = cur->next;
}
if (cur == NULL) { // 如果没有找到,则插入失败
cout << "插入失败,位置不存在!" << endl;
return;
}
ListNode* newNode = new ListNode(x); // 创建新结点
newNode->next = cur->next; // 将新结点链接到当前结点的后面
cur->next = newNode; // 将当前结点链接到新结点后面
}
// 从链表中删除一个元素
void deleteElement(ListNode* head, int x) {
ListNode* cur = head; // 从头结点开始查找
while (cur->next != NULL && cur->next->val != x) { // 找到要删除的结点的前一个结点
cur = cur->next;
}
if (cur->next == NULL) { // 如果没有找到,则删除失败
cout << "删除失败,元素不存在!" << endl;
return;
}
ListNode* temp = cur->next; // 找到要删除的结点
cur->next = temp->next; // 将要删除的结点从链表中删除
delete temp; // 释放要删除的结点的空间
}
// 遍历链表并输出所有元素
void traverseList(ListNode* head) {
ListNode* cur = head->next; // 从第一个结点开始遍历
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
int main() {
ListNode* head = createList(); // 建立链表
traverseList(head); // 输出链表元素
int x, pos;
cout << "请输入要查找的元素:";
cin >> x;
if (findElement(head, x)) {
cout << "元素 " << x << " 存在!" << endl;
} else {
cout << "元素 " << x << " 不存在!" << endl;
}
cout << "请输入要插入的元素和位置(位置从0开始):";
cin >> x >> pos;
insertElement(head, pos, x);
traverseList(head);
cout << "请输入要删除的元素:";
cin >> x;
deleteElement(head, x);
traverseList(head);
return 0;
}
```
程序中,我们首先定义了一个链表节点结构体 `ListNode`,其中包含一个整数值 `val` 和一个指向下一个节点的指针 `next`。然后,我们实现了链表的建立、查找、插入、删除和遍历等基本操作。在主函数中,我们通过调用这些函数来对链表进行操作,从而实现了整数单链表的建立、查找、插入、删除和输出。
阅读全文
相关推荐
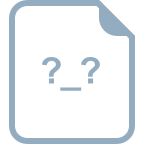
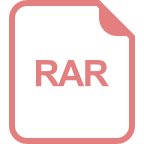





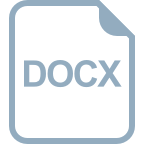
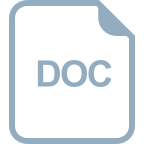
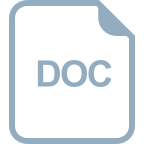
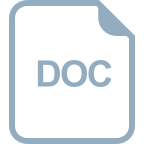
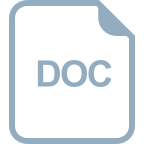
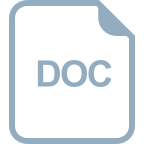
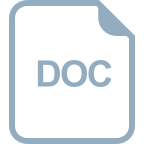
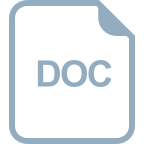
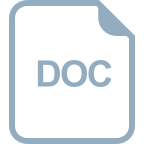
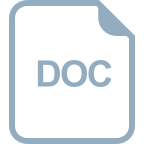
