请用C++写一个整数单链表的建立、查找、插入、删除、输出程序(线性表的链式存储实现),表中不允许有重复数据,保存为LinkList文件。
时间: 2023-10-19 15:21:34 浏览: 112
好的,以下是代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
void createList(Node **head, int n); // 建立单链表
void traverseList(Node *head); // 遍历单链表并输出每个节点的值
int searchList(Node *head, int value); // 查找单链表中是否存在某个值
void insertList(Node **head, int value); // 在单链表中插入某个值
void deleteList(Node **head, int value); // 在单链表中删除某个值
int main() {
Node *head = NULL;
int n, value, pos;
printf("请输入链表的长度:");
scanf("%d", &n);
createList(&head, n);
traverseList(head);
printf("\n请输入要查找的值:");
scanf("%d", &value);
pos = searchList(head, value);
if (pos == -1) {
printf("未找到该值\n");
} else {
printf("该值在链表中的位置为:%d\n", pos);
}
printf("\n请输入要插入的值:");
scanf("%d", &value);
insertList(&head, value);
traverseList(head);
printf("\n请输入要删除的值:");
scanf("%d", &value);
deleteList(&head, value);
traverseList(head);
return 0;
}
void createList(Node **head, int n) {
Node *p, *tail;
int i, value;
*head = (Node *) malloc(sizeof(Node));
(*head)->next = NULL;
tail = *head;
for (i = 1; i <= n; i++) {
printf("请输入第%d个节点的值:", i);
scanf("%d", &value);
if (searchList(*head, value) == -1) {
p = (Node *) malloc(sizeof(Node));
p->data = value;
p->next = NULL;
tail->next = p;
tail = p;
} else {
printf("该值已存在,请重新输入\n");
i--;
}
}
}
void traverseList(Node *head) {
Node *p = head->next;
printf("链表的值为:");
while (p) {
printf("%d ", p->data);
p = p->next;
}
}
int searchList(Node *head, int value) {
Node *p = head->next;
int pos = 1;
while (p) {
if (p->data == value) {
return pos;
}
p = p->next;
pos++;
}
return -1;
}
void insertList(Node **head, int value) {
Node *p, *q;
q = *head;
if (searchList(*head, value) != -1) {
printf("该值已存在,无需插入\n");
return;
}
while (q->next) {
if (q->next->data > value) {
break;
}
q = q->next;
}
p = (Node *) malloc(sizeof(Node));
p->data = value;
p->next = q->next;
q->next = p;
printf("插入成功\n");
}
void deleteList(Node **head, int value) {
Node *p, *q;
q = *head;
while (q->next) {
if (q->next->data == value) {
p = q->next;
q->next = p->next;
free(p);
printf("删除成功\n");
return;
}
q = q->next;
}
printf("未找到该值\n");
}
```
该程序实现了单链表的建立、查找、插入、删除和输出功能。其中,建立单链表时,程序会提示用户输入每个节点的值,并检查该值是否已存在于链表中;查找时,程序会输出该值在链表中的位置;插入时,程序会按照从小到大的顺序插入该值,并检查该值是否已存在于链表中;删除时,程序会删除链表中第一个等于该值的节点。
阅读全文
相关推荐



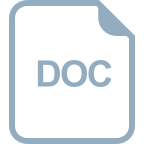
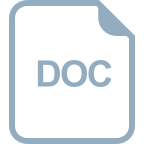


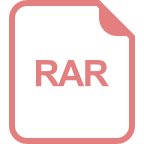
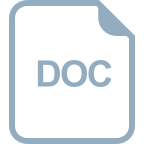
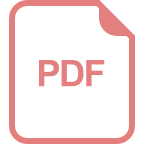
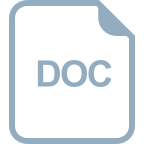
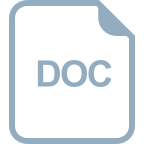
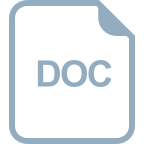


