实现线性表的链式存储结构c语言
时间: 2024-06-15 13:09:50 浏览: 124
在C语言中,实现线性表的链式存储结构可以使用指针来实现。链式存储结构是通过节点之间的指针连接来表示线性表中的元素关系。
首先,我们需要定义一个节点结构体,该结构体包含两个成员:数据域和指针域。数据域用于存储节点中的数据,指针域用于指向下一个节点。
```c
// 定义节点结构体
typedef struct Node {
int data; // 数据域
struct Node* next; // 指针域,指向下一个节点
} Node;
```
接下来,我们可以定义一个链表结构体,该结构体包含一个指向头节点的指针。
```c
// 定义链表结构体
typedef struct LinkedList {
Node* head; // 头节点指针
} LinkedList;
```
然后,我们可以实现一些基本的操作函数来对链表进行操作,例如插入、删除、查找等。
1. 插入操作:在链表中插入一个新节点,可以在头部插入或者在指定位置插入。
```c
// 在头部插入新节点
void insertAtHead(LinkedList* list, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = list->head;
list->head = newNode;
}
// 在指定位置插入新节点
void insertAtPosition(LinkedList* list, int data, int position) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
if (position == 0) {
newNode->next = list->head;
list->head = newNode;
} else {
Node* current = list->head;
for (int i = 0; i < position - 1 && current != NULL; i++) {
current = current->next;
}
if (current != NULL) {
newNode->next = current->next;
current->next = newNode;
}
}
}
```
2. 删除操作:从链表中删除指定位置的节点。
```c
// 删除指定位置的节点
void deleteAtPosition(LinkedList* list, int position) {
if (list->head == NULL) {
return;
}
Node* temp = list->head;
if (position == 0) {
list->head = temp->next;
free(temp);
return;
}
for (int i = 0; temp != NULL && i < position - 1; i++) {
temp = temp->next;
}
if (temp == NULL || temp->next == NULL) {
return;
}
Node* nextNode = temp->next->next;
free(temp->next);
temp->next = nextNode;
}
```
3. 查找操作:在链表中查找指定值的节点。
```c
// 查找指定值的节点
Node* search(LinkedList* list, int value) {
Node* current = list->head;
while (current != NULL) {
if (current->data == value) {
return current;
}
current = current->next;
}
return NULL;
}
```
这样,我们就可以使用上述定义的结构体和函数来实现线性表的链式存储结构了。
阅读全文
相关推荐

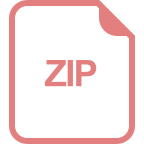
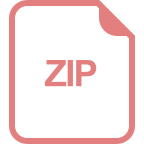
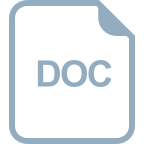
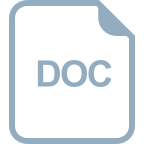




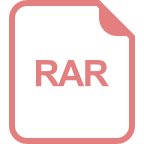
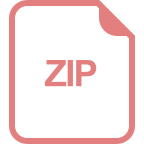
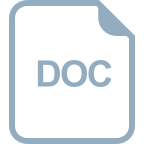
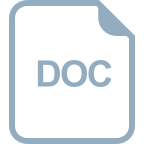
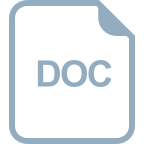