线性表的链式实现c语言版csdn
时间: 2023-10-22 12:01:27 浏览: 63
链式实现线性表的C语言版本可以通过定义一个结构体来表示链表节点,结构体中包含存储的数据以及指向下一个节点的指针。具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data; // 数据域
struct ListNode *next; // 指针域,指向下一个节点
} ListNode;
// 创建链表节点
ListNode* createNode(int value) {
ListNode *node = (ListNode*) malloc(sizeof(ListNode));
node->data = value;
node->next = NULL;
return node;
}
// 插入节点到链表尾部
void insert(ListNode **head, int value) {
ListNode *node = createNode(value);
if (*head == NULL) {
*head = node;
} else {
ListNode *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = node;
}
}
// 打印链表
void printList(ListNode *head) {
ListNode *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
ListNode *head = NULL;
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
printList(head); // 输出:1 2 3
return 0;
}
```
以上代码中,通过`createNode`函数创建一个新的节点,并返回该节点的指针。`insert`函数用于将节点插入链表的末尾,如果链表为空,则直接将该节点赋值给链表的头指针,否则找到链表的末尾节点,将其next指针指向新节点。`printList`函数用于遍历链表并打印每个节点的数据。在`main`函数中,通过调用`insert`函数将数据插入链表,然后通过调用`printList`函数打印链表的数据。
相关推荐
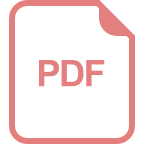
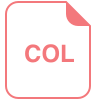
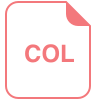
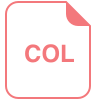
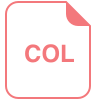
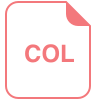









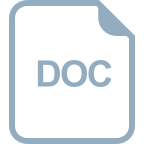