数据结构线性表查找代码,用c语言写,不要引用外部函数库,用关键词算法进行查找
时间: 2024-03-09 21:46:04 浏览: 111
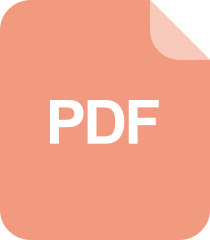
c语言实现线性表的算法-数据结构算法代码实现——线性表的定义(一) 定义线性表节点的结构.pdf
以下是一个用C语言写的线性表查找代码,不引用外部函数库,使用关键词算法进行查找:
```c
#include <stdio.h>
#define MAX_SIZE 100 // 线性表最大长度
struct List {
int data[MAX_SIZE]; // 存储元素的数组
int length; // 线性表当前长度
};
// 初始化一个空的线性表
void initList(struct List *L) {
L->length = 0;
}
// 在线性表的末尾插入一个元素
void insert(struct List *L, int x) {
if (L->length >= MAX_SIZE) {
printf("Error: Linear list is full!\n");
return;
}
L->data[L->length] = x;
L->length++;
}
// 在线性表中查找值为x的元素,返回其下标,如果找不到则返回-1
int search(struct List L, int x) {
for (int i = 0; i < L.length; i++) {
if (L.data[i] == x) {
return i;
}
}
return -1;
}
int main() {
struct List L;
initList(&L);
insert(&L, 1);
insert(&L, 2);
insert(&L, 3);
int x;
printf("Please enter the value you want to search: ");
scanf("%d", &x);
int index = search(L, x);
if (index == -1) {
printf("The value %d is not found in the linear list.\n", x);
} else {
printf("The value %d is found at index %d in the linear list.\n", x, index);
}
return 0;
}
```
该代码与前面的C++代码类似,只是语法和部分细节有所不同。我们使用结构体List来表示线性表,使用指针来操作结构体。initList和insert函数不改变List结构体本身,所以我们可以使用指针来传递结构体。search函数使用for循环遍历线性表,使用if语句进行查找。
阅读全文
相关推荐
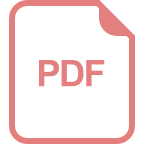
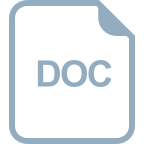
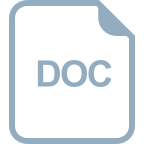
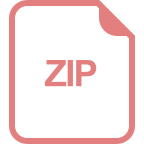
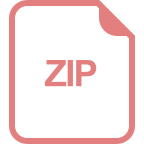
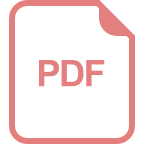
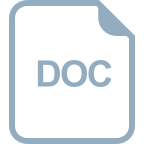
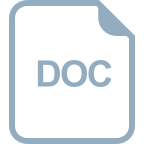
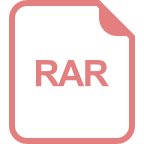
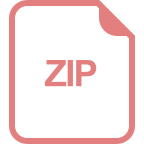
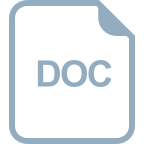
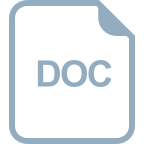
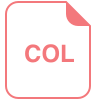
