linux如何调用USB串口设备文件
时间: 2023-12-13 20:02:55 浏览: 35
在Linux中,USB串口设备文件通常命名为/dev/ttyUSBx,其中x为设备号。要调用一个USB串口设备文件,您需要先确定您的设备号,然后使用串口通信程序(如minicom、cu、picocom等)或编程语言(如C、Python等)打开该设备文件。以下是一个使用minicom打开USB串口设备的示例命令:
```
minicom -D /dev/ttyUSB0
```
其中,/dev/ttyUSB0是您的USB串口设备文件。您可以将其替换为您自己的设备文件路径。此命令将打开一个minicom终端,您可以在终端中发送和接收数据。
如果您想在编程中调用USB串口设备文件,可以使用POSIX标准的串口API(如open、read、write、close等)或相关的库(如libserial、pyserial等)来访问设备文件。例如,在C语言中,您可以使用以下代码打开一个USB串口设备文件:
```C
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY);
if (fd == -1)
{
perror("open");
return -1;
}
//设置串口属性
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
//读写数据
char buf[100];
int n = read(fd, buf, sizeof(buf));
printf("read %d bytes: %s\n", n, buf);
n = write(fd, "hello", 5);
printf("write %d bytes\n", n);
//关闭设备文件
close(fd);
return 0;
}
```
该程序将打开一个名为/dev/ttyUSB0的USB串口设备文件,并设置串口属性为9600波特率、8数据位、无校验位、1停止位,然后读取设备文件中的数据并写入数据。您可以根据自己的需求修改代码。
相关推荐
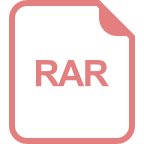
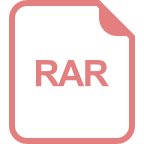
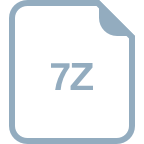














