编译下列程序,改正所出现的错误信息,并写出输出结果。 1) //sy1_1.cpp main() { cout<<”This is a program.” } 2) //sy1_2.cpp #include<iostream> using namespace std; int main() { cin>>x; int y=x*x; cout<<”y=<<y<<\n”; return 0; } 3) //sy1_3.cpp #include<iostream> using namespace std; int main() { int a,b; a=7; int s=a+b; cout<<”a+b”<<s<<endl; return 0; }
时间: 2024-02-27 16:57:07 浏览: 21
1) //sy1_1.cpp
```
#include<iostream>
using namespace std;
int main() {
cout<<"This is a program.";
return 0;
}
```
输出结果为:
This is a program.
2) //sy1_2.cpp
```
#include<iostream>
using namespace std;
int main() {
int x;
cin>>x;
int y=x*x;
cout<<"y="<<y<<"\n";
return 0;
}
```
在输入一个整数后,输出结果为该整数的平方值。
3) //sy1_3.cpp
```
#include<iostream>
using namespace std;
int main() {
int a=7,b=9;
int s=a+b;
cout<<"a+b="<<s<<endl;
return 0;
}
```
输出结果为:
a+b=16
相关问题
APM编译[ 92/509] Compiling libraries/AP_Compass/AP_Compass_Calibration.cpp报错
您好!对于您提到的错误,可能有几种解决方法。首先,您可以检查编译器输出的详细错误信息,以确定出现问题的具体位置和原因。此外,您还可以尝试以下几种方法来解决该问题:
1. 检查代码:请确保您的代码没有语法错误或逻辑错误。特别是查看 `AP_Compass_Calibration.cpp` 文件中的代码,看是否存在错误或者遗漏的分号等。
2. 检查依赖项:编译过程中可能需要一些依赖项或库文件。请确保您的环境中已经正确安装了这些依赖项,并且路径设置正确。
3. 更新编译器:尝试更新您使用的编译器版本。有时,旧版本的编译器可能存在一些问题,更新到最新版本可能会解决这些问题。
4. 检查编译选项:检查您使用的编译选项是否正确设置。有时,编译选项可能会导致编译错误。
5. 查找解决方案:在开发社区或相关论坛中搜索类似的问题。其他开发者可能已经遇到过相似的问题,并提供了解决方案。
希望以上方法能对您有所帮助!如果问题仍然存在,请提供更多详细信息,我将尽力提供更准确的解决方案。
/home/share/rv1126_sdk_2_2_4/external/mpp/osal/mpp_soc.cpp:19:
### 回答1:
这是一段错误信息,指出在路径"/home/share/rv1126_sdk_2_2_4/external/mpp/osal/mpp_soc.cpp"的第19行发生了错误。
根据路径结构,这是一段代码文件mpp_soc.cpp,位于路径"/home/share/rv1126_sdk_2_2_4/external/mpp/osal/"下。
错误信息没有具体说明错误的性质,可能是编译错误、语法错误、逻辑错误等等。想要解决这个错误,我们需要查看相应的代码文件并检查第19行附近的代码。
可能的解决方法包括:
1. 检查第19行的代码,确认是否有语法错误、拼写错误等问题。
2. 检查变量、函数或方法是否正确声明和调用,确保其存在和正确使用。
3. 查找相关的编译或运行日志,以获取更多关于错误的信息,有助于确定出错原因。
4. 如果有编译选项或相关配置文件,检查其是否正确设置。
5. 可以尝试使用调试器逐行调试代码,以确定错误发生的具体位置和原因。
总结起来,对于这个错误信息,我们需要检查并排除代码中的错误,找出根本原因并进行相应的修复。
### 回答2:
"/home/share/rv1126_sdk_2_2_4/external/mpp/osal/mpp_soc.cpp:19" 是一个文件路径和行号的错误信息提示。该提示表明在指定的路径中的 "mpp_soc.cpp" 文件的第19行出现了错误。
根据错误提示的信息,我们可以推测这个错误很可能与文件中的代码有关。可能是代码中存在语法错误、逻辑错误或者其他类型的错误导致了程序运行失败。
要解决这个错误,首先需要定位到 "mpp_soc.cpp" 文件的第19行,进一步分析代码,检查是否存在拼写错误、缺少分号、参数不匹配等常见的语法错误。还可以通过调试器逐步执行代码,观察错误发生的具体情况,进一步定位问题所在。
此外,还可以查看错误信息中提到的文件路径是否正确,有时候错误可能是由于文件路径不正确导致的。可以检查该路径是否存在、拼写是否正确,并确保程序有访问该路径的权限。
最后,如果以上方法都无法解决问题,可以尝试搜索相关的错误提示信息,查找相关的解决方案或者寻求开发者社区的帮助,以解决该错误。
### 回答3:
/home/share/rv1126_sdk_2_2_4/external/mpp/osal/mpp_soc.cpp:19: 是一个文件路径,它表示在rv1126_sdk_2_2_4目录下的external/mpp/osal目录中的mpp_soc.cpp文件的第19行。
这个文件路径告诉我们,这是一个文件在计算机文件系统中的位置。文件路径的组成部分是目录名和文件名,在这个路径中,我们可以看到rv1126_sdk_2_2_4是根目录,它下面有一个external目录,再下面有一个mpp目录,最后是osal目录。在osal目录中,有一个名为mpp_soc.cpp的文件。:19表示文件的第19行。
文件路径的用途是唯一标识文件的位置,通过文件路径,我们可以准确定位到计算机文件系统中的一个特定文件。对于程序员来说,文件路径非常重要,因为它指导了他们在编程时需要操作的文件的位置。在这个路径中,我们可以看到这个文件位于一个SDK的外部目录,这意味着该文件可能是SDK中用于操作硬件设备的一部分。
在这个特定的路径中,文件应该是一个名为mpp_soc.cpp的源代码文件。通过这个文件的路径和行号,程序员可以准确地定位到代码中的某一行,以便调试和修改代码错误。
总之,/home/share/rv1126_sdk_2_2_4/external/mpp/osal/mpp_soc.cpp:19: 表示文件路径为/home/share/rv1126_sdk_2_2_4/external/mpp/osal/mpp_soc.cpp,要操作的文件为mpp_soc.cpp,位于该文件的第19行。
相关推荐
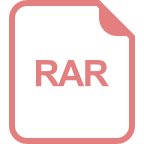
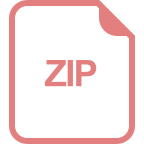













