C++在vector中存入整数,判断是否为回文数
时间: 2024-02-11 22:04:46 浏览: 44
可以通过以下步骤来判断一个vector中存储的整数是否为回文数:
1. 将整数按照位数拆分,并将每一位存入vector中,例如将整数12321拆分为{1, 2, 3, 2, 1}。
2. 使用双指针法,从vector的两端开始向中间遍历,依次比较对应位置上的数字是否相同,如果有不同的则说明该整数不是回文数,反之则为回文数。
以下是C++代码实现:
```c++
#include <iostream>
#include <vector>
using namespace std;
bool isPalindrome(vector<int>& nums) {
int left = 0, right = nums.size() - 1;
while (left < right) {
if (nums[left] != nums[right]) {
return false;
}
left++;
right--;
}
return true;
}
int main() {
int n;
vector<int> nums;
cin >> n;
while (n > 0) {
nums.push_back(n % 10);
n /= 10;
}
bool res = isPalindrome(nums);
if (res) {
cout << "Yes" << endl;
} else {
cout << "No" << endl;
}
return 0;
}
```
相关推荐
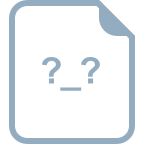
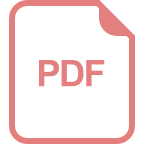
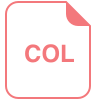
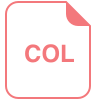
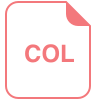
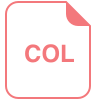
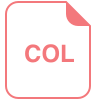









