bp神经网络分类mnist数据集
时间: 2024-12-13 09:12:36 浏览: 4
BP(Back Propagation)神经网络是一种常用的前馈神经网络,主要用于分类和回归问题。MNIST数据集是一个手写数字识别的经典数据集,包含60000张训练图片和10000张测试图片,每张图片是28x28像素的灰度图,标注为0-9的数字。
使用BP神经网络对MNIST数据集进行分类的步骤如下:
1. **数据预处理**:
- 将图像数据归一化:将像素值从[0, 255]范围缩放到[0, 1]范围。
- 将标签进行独热编码(One-Hot Encoding),即将每个标签转换为一个10维的向量,例如标签3转换为[0, 0, 0, 1, 0, 0, 0, 0, 0, 0]。
2. **构建BP神经网络**:
- 输入层:输入层的节点数与图像的像素数相同,即28x28=784。
- 隐藏层:可以设置一个或多个隐藏层,每层的节点数可以根据需要调整。
- 输出层:输出层的节点数为10,对应于10个类别。
3. **训练网络**:
- 初始化权重和偏置。
- 前向传播:计算每层的输出。
- 计算损失:通常使用交叉熵损失函数。
- 反向传播:计算损失函数对每个参数的梯度,并更新权重和偏置。
4. **评估模型**:
- 使用测试集评估模型的准确率。
以下是一个简单的Python代码示例,使用TensorFlow和Keras库来实现BP神经网络对MNIST数据集的分类:
```python
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten
from tensorflow.keras.utils import to_categorical
# 加载数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape((x_train.shape[0], 28 * 28)) / 255.0
x_test = x_test.reshape((x_test.shape[0], 28 * 28)) / 255.0
y_train = to_categorical(y_train, 10)
y_test = to_categorical(y_test, 10)
# 构建BP神经网络
model = Sequential()
model.add(Flatten(input_shape=(28 * 28,)))
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=10, batch_size=32, validation_split=0.1)
# 评估模型
test_loss, test_accuracy = model.evaluate(x_test, y_test)
print(f"Test accuracy: {test_accuracy}")
```
阅读全文
相关推荐
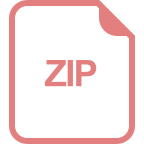
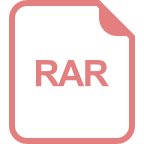
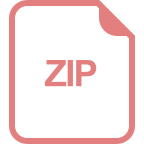

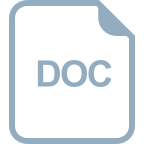
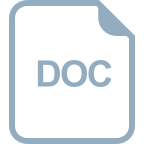
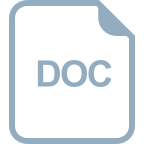
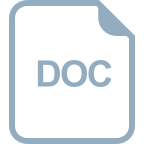
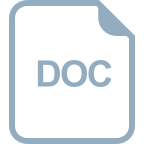










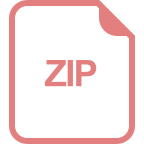