在julia不使用Flux编写一个含有一层隐含层的神经网络
时间: 2024-03-17 10:46:38 浏览: 18
好的,以下是在Julia中不使用Flux编写一个含有一层隐含层的神经网络的示例代码:
```julia
# 定义sigmoid函数
sigmoid(x) = 1 / (1 + exp(-x))
# 定义神经网络模型
struct NeuralNetwork
W1
W2
b1
b2
end
# 定义模型的前向传播函数
function predict(model, x)
z1 = model.W1 * x .+ model.b1
a1 = sigmoid.(z1)
z2 = model.W2 * a1 .+ model.b2
y = softmax(z2)
return y
end
# 定义损失函数
function loss(y_pred, y_true)
return -sum(y_true .* log.(y_pred))
end
# 定义优化器
function update!(model, lr, grads)
model.W1 -= lr * grads[1]
model.W2 -= lr * grads[2]
model.b1 -= lr * grads[3]
model.b2 -= lr * grads[4]
end
# 定义训练函数
function train(model, x, y_true, lr)
# 前向传播
y_pred = predict(model, x)
# 计算损失
loss_value = loss(y_pred, y_true)
# 反向传播
grads = gradients(loss_value, params(model))
# 更新参数
update!(model, lr, grads)
return loss_value
end
# 测试模型
function test(model, x, y_true)
y_pred = predict(model, x)
accuracy = sum(argmax(y_pred, dim=1) .== argmax(y_true, dim=1)) / size(y_true, 2)
return accuracy
end
# 初始化模型
function init_model(input_size, hidden_size, output_size)
W1 = randn(hidden_size, input_size)
W2 = randn(output_size, hidden_size)
b1 = zeros(hidden_size, 1)
b2 = zeros(output_size, 1)
model = NeuralNetwork(W1, W2, b1, b2)
return model
end
# 定义训练数据
x_train = rand(4, 1000)
y_train = rand(3, 1000)
# 初始化模型
model = init_model(4, 5, 3)
# 训练模型
for i in 1:1000
loss_value = train(model, x_train, y_train, 0.1)
if i % 100 == 0
@show loss_value
end
end
# 测试模型
x_test = rand(4, 100)
y_test = rand(3, 100)
accuracy = test(model, x_test, y_test)
@show accuracy
```
在这个示例中,我们手动定义了模型的参数和前向传播函数,并使用自己实现的梯度计算和优化器来进行训练。虽然这种方法比使用Flux更加麻烦,但也更加灵活,可以更好地理解神经网络的内部运作。
相关推荐
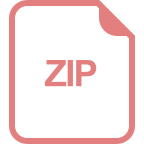
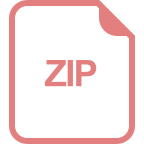
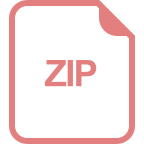














