编写程序,读取 Word 文件中的所有段落文本,然后输出其中所有红色的文本和加粗的文本以及同时具有这两种属性的文本
时间: 2024-12-22 11:30:06 浏览: 9
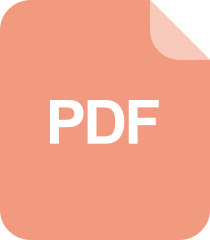
matlab 中如何读取文本文件.pdf
为了实现这个功能,你需要使用Python的`python-docx`库来读取Word文档,并利用`docx`对象的`paragraphs`属性获取所有的段落。对于颜色和字体样式,`python-docx`库并不直接支持颜色检测,但可以通过第三方库如`olefile`来解析Word的二进制数据找到字体信息。以下是一个简单的示例,展示如何使用`python-docx`和`olefile`来达到目标,但这可能会有些复杂:
```python
from docx import Document
from olefile import OleFileIO
from collections import defaultdict
# 定义字体样式和颜色的字典
styles_and_colors = {
"bold": (1, "font-bold"),
"red": (4, "font-color-red"),
# 添加更多样式...
}
def process_word_file(filename):
document = Document(filename)
paragraphs_with_styles = defaultdict(list)
for paragraph in document.paragraphs:
styles = paragraph.style.font.Styles
color_index = -1
bold_index = -1
# 查找红色或加粗的字体
for style_id, style_name in styles_and_colors.items():
if style_id in styles:
if style_name == "font-color-red":
color_index = styles.index(style_id)
elif style_name == "font-bold":
bold_index = styles.index(style_id)
# 如果找到了,添加到列表中
if color_index >= 0 and bold_index >= 0:
paragraphs_with_styles[(color_index, bold_index)].append(paragraph.text)
result = {}
for key, text_list in paragraphs_with_styles.items():
red_bold_text = ", ".join(text_list)
result[f"红色并加粗({styles_and_colors[key][1]})"] = red_bold_text
return result
# 使用函数并打印结果
output = process_word_file("example.docx")
for key, value in output.items():
print(f"{key}: {value}")
```
注意:这个代码片段依赖于`python-docx`和`olefile`库,如果你还没有安装,可以使用pip进行安装:
```bash
pip install python-docx olefile
```
然而,这个过程可能非常消耗资源,因为Word文件的解析通常不是实时的,并且`olefile`库并不是特别适合这种频繁的文件操作。
阅读全文
相关推荐
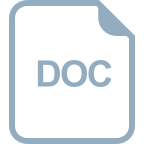
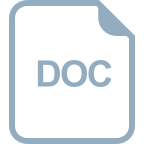




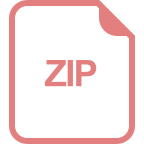
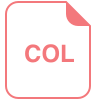
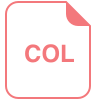
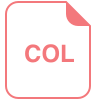
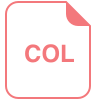
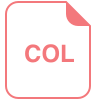
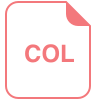
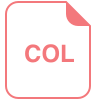
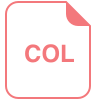
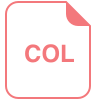
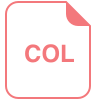