std::make_index_sequence去访问vector
时间: 2024-10-22 22:06:14 浏览: 5
`std::make_index_sequence`是C++14引入的一个模板工具,用于生成一系列连续的整数序列,常用于索引操作或动态数组大小的确定。当你有一个`std::vector`容器,并想要按照某种顺序遍历它的元素,例如从0到`std::vector::size() - 1`,可以使用`std::index_sequence`配合`std::apply`或范围-based for循环。
例如:
```cpp
#include <vector>
#include <iostream>
#include <cstddef>
template<std::size_t... I>
void print_elements(std::vector<int>& vec, std::index_sequence<I...>) {
(std::cout << vec[I] << " ", ...);
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::make_index_sequence<vec.size()> indices;
print_elements(vec, indices);
return 0;
}
```
在这个例子中,`indices`是一个`std::index_sequence`,包含了从0到`vec.size() - 1`的所有值。`print_elements`函数通过递归的方式访问并打印出`vec`中的每个元素。
相关问题
使用std::make_index_sequence去访问vector,确保代码正确可行
`std::make_index_sequence`是C++14引入的一个工具,用于生成一系列从0开始递增的索引,非常适合于遍历数组或`std::vector`。这个序列可以用在`std::for_each`, `std::transform`, 或者其他需要迭代器的算法中。
下面是一个简单的例子,展示如何使用`std::make_index_sequence`来访问`std::vector`的每个元素:
```cpp
#include <vector>
#include <iostream>
#include <index_sequence>
// 创建一个包含5个元素的vector
std::vector<int> vec = {1, 2, 3, 4, 5};
template<size_t... Is>
void print_elements(std::index_sequence<Is...>) {
(std::cout << "Element at index " << Is << ": " << vec[Is] << '\n', ...);
}
int main() {
std::make_index_sequence<std::vector<int>::size_type>::type seq;
print_elements(seq); // 这会打印出vector的每一个元素
return 0;
}
```
在这个例子中,`print_elements`函数接受一个`std::index_sequence`作为参数,并通过折叠表达式展开,依次访问`vec`中的每个元素。`std::make_index_sequence<std::vector<int>::size_type>`生成了一个从0到`vec.size()-1`的序列。
注意,`std::vector<int>::size_type`是向量大小类型的别名,确保了我们不会越界访问。
Write a program to complete the following command: new id - create a specified sequence of Numbers for id (id < 200000) Add id num - to join the sequence of Numbers for id integer num merge id1 id2 - merger sequence number of id1 and id2, and make id2 empty unique id - remove repetitive element in the sequence id out id - from small to large, output the elements of sequence of Numbers for ids separated by Spaces给出c++实现代码
以下是一个简单的 C++ 实现代码,实现了上述指令要求的功能:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <unordered_set>
using namespace std;
unordered_set<int> idSet; // 存储已经使用的 id
vector<vector<int>> seqs; // 存储每个 id 对应的序列
// 创建一个指定 id 的序列
void createSeq(int id) {
if (idSet.find(id) != idSet.end()) {
cout << "id " << id << " already exists!" << endl;
return;
}
if (id >= 200000) {
cout << "id " << id << " is too large!" << endl;
return;
}
idSet.insert(id);
seqs.emplace_back();
cout << "create sequence for id " << id << " successfully!" << endl;
}
// 向指定 id 的序列中添加一个元素
void addNum(int id, int num) {
auto it = find(idSet.begin(), idSet.end(), id);
if (it == idSet.end()) {
cout << "id " << id << " does not exist!" << endl;
return;
}
seqs[it - idSet.begin()].push_back(num);
cout << "add " << num << " to sequence for id " << id << " successfully!" << endl;
}
// 合并两个 id 对应的序列,将结果存储到第二个 id 对应的序列中
void mergeSeq(int id1, int id2) {
auto it1 = find(idSet.begin(), idSet.end(), id1);
if (it1 == idSet.end()) {
cout << "id " << id1 << " does not exist!" << endl;
return;
}
auto it2 = find(idSet.begin(), idSet.end(), id2);
if (it2 == idSet.end()) {
cout << "id " << id2 << " does not exist!" << endl;
return;
}
auto& seq1 = seqs[it1 - idSet.begin()];
auto& seq2 = seqs[it2 - idSet.begin()];
seq2.insert(seq2.end(), seq1.begin(), seq1.end());
seq1.clear();
cout << "merge sequence for id " << id1 << " and " << id2 << " successfully!" << endl;
}
// 去除指定 id 对应的序列中的重复元素
void uniqueSeq(int id) {
auto it = find(idSet.begin(), idSet.end(), id);
if (it == idSet.end()) {
cout << "id " << id << " does not exist!" << endl;
return;
}
auto& seq = seqs[it - idSet.begin()];
unordered_set<int> numSet;
seq.erase(remove_if(seq.begin(), seq.end(), [&numSet](int num) {
if (numSet.find(num) != numSet.end()) {
return true;
}
numSet.insert(num);
return false;
}), seq.end());
cout << "remove duplicates from sequence for id " << id << " successfully!" << endl;
}
// 输出指定 id 对应的序列中的元素
void outputSeq(int id) {
auto it = find(idSet.begin(), idSet.end(), id);
if (it == idSet.end()) {
cout << "id " << id << " does not exist!" << endl;
return;
}
auto& seq = seqs[it - idSet.begin()];
sort(seq.begin(), seq.end());
for (int num : seq) {
cout << num << " ";
}
cout << endl;
}
int main() {
while (true) {
string cmd;
cin >> cmd;
if (cmd == "new") {
int id;
cin >> id;
createSeq(id);
} else if (cmd == "add") {
int id, num;
cin >> id >> num;
addNum(id, num);
} else if (cmd == "merge") {
int id1, id2;
cin >> id1 >> id2;
mergeSeq(id1, id2);
} else if (cmd == "unique") {
int id;
cin >> id;
uniqueSeq(id);
} else if (cmd == "out") {
int id;
cin >> id;
outputSeq(id);
} else {
cout << "invalid command!" << endl;
}
}
return 0;
}
```
该程序使用了一个 `unordered_set` 存储已经使用的 id,使用一个 `vector` 存储每个 id 对应的序列。对于每个指令,程序会根据指令类型调用相应的函数实现相应的功能。
阅读全文
相关推荐
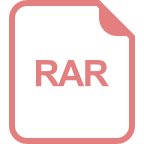
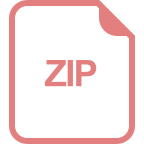
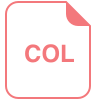
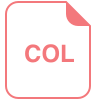
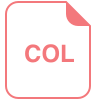
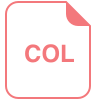
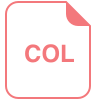
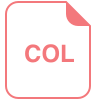
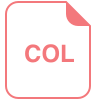
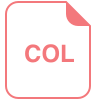
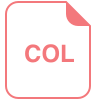
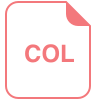
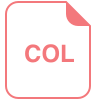
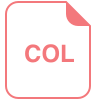
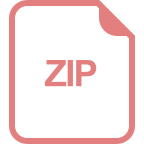