写一个调用yolov8的程序
时间: 2024-09-21 12:12:36 浏览: 43
YOLOv8 (You Only Look Once version 8) 是一种流行的实时目标检测算法,基于开源项目 Darknet 开发。要在 Python 中编写一个简单的 YOLOv8 程序,你需要安装必要的库,如 OpenCV、PyTorch及其Dlib等,并加载预训练模型。
以下是一个基本的步骤示例:
```python
import cv2
import torch
# 安装必要的库(如果你还没安装)
!pip install opencv-python-headless torchvision yolo-v8-pytorch darkflow
from models.experimental import attempt_load
from utils.datasets import LoadStreams, LoadImages
from utils.general import non_max_suppression, check_img_size
# 加载预训练模型
model = attempt_load('yolov8.weights', map_location='cuda:0') if torch.cuda.is_available() else attempt_load('yolov8.weights', map_location='cpu')
# 设置输入大小
imgsz = 640
# 使用YoloV8进行实时检测
def detect_objects(streams, imgsz):
for path, im in streams:
try:
img = preprocess_image(im, imgsz)
results = model(img)
output = non_max_suppression(results, conf_thres=0.5, iou_thres=0.5)
draw_output(path, im, output)
except Exception as e:
print(f"Error during detection: {e}")
def preprocess_image(image, size):
# 调整图像尺寸并进行前处理
img = cv2.resize(image, (size, size))
img = img[:, :, ::-1].transpose(2, 0, 1) # BGR to RGB and HWC to CHW
img = np.ascontiguousarray(img)
return img
def draw_output(path, image, boxes):
# 绘制预测结果到原图上
for result in boxes:
box, label, prob = result
cv2.rectangle(image, (box[0], box[1]), (box[2], box[3]), (255, 0, 0), 2)
cv2.putText(image, f'{label}: {prob:.2f}', (box[0] + 20, box[1] - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
# 初始化数据加载器
streams = LoadImages('your_video.mp4') # 或者LoadStreams('path/to/images/*.jpg', img_size=imgsz)
# 开始实时检测
detect_objects(streams, imgsz)
```
注意替换 `your_video.mp4` 或 `'path/to/images/*.jpg'` 为你实际视频文件路径或图片目录。这个脚本将读取视频流并对其中的目标进行实时检测。
阅读全文
相关推荐
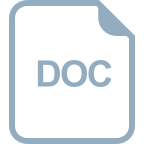
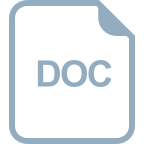
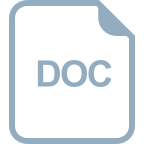
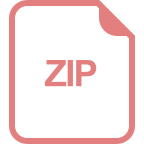
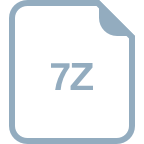
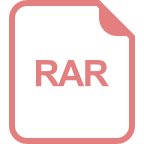
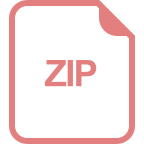











