python怎么启动一个线程并在需要的时候手动终止它
时间: 2024-10-27 16:05:36 浏览: 7
在Python中,你可以使用`threading`模块来创建并控制线程。下面是一个简单的例子展示了如何启动线程和如何使用`Thread`类的`join()`方法来手动终止线程:
```python
import threading
import time
# 定义一个函数作为线程的任务
def worker_thread():
print("Worker thread started")
while True:
if stop_event.is_set(): # 使用一个事件来检查是否需要停止
print("Worker thread stopping")
break
time.sleep(1) # 模拟工作过程
# 初始化一个事件来标志是否需要停止
stop_event = threading.Event() # 默认关闭,即线程一开始不会停止
# 创建一个新的线程
worker_thread_obj = threading.Thread(target=worker_thread)
# 启动线程
worker_thread_obj.start()
print("Worker thread is running")
# 当需要时,设置stop_event
stop_event.set()
# 等待线程完成任务
worker_thread_obj.join()
print("Worker thread stopped")
相关问题
python多线程中终止线程
在Python中,如果你想要终止一个正在运行的线程,你可以通过`Thread`对象的`terminate()`、`join()`或`cancel()`方法来实现。以下是几种常见的方法:
1. **terminate()**: 这个方法会发送一个SIGTERM信号给线程,请求其停止。如果线程还没有处理这个信号,它会在一定时间后强制退出。例如:
```python
import threading
def target_function():
# 线程代码...
thread = threading.Thread(target=target_function)
# ...启动线程...
# 要终止线程
if thread.is_alive(): # 检查线程是否还在运行
thread.terminate()
```
请注意,这并不是一种优雅的方式来结束线程,因为可能会导致数据未保存等异常。
2. **join()**: 使用`join()`方法可以等待线程执行完毕再继续。如果设定了超时时间,可以通过`thread.join(timeout)`来在指定秒数内等待线程结束。如果超时,线程不会被终止,而是返回到主线程。
```python
thread.join() # 如果设置timeout参数,如thread.join(5),那么最多等待5秒
```
3. **cancel()**: 在Windows平台上,你可以使用`Event`对象配合`ThreadPoolExecutor`来取消任务,但它不是所有平台通用的。例如:
```python
from concurrent.futures import ThreadPoolExecutor
with ThreadPoolExecutor(max_workers=1) as executor:
future = executor.submit(target_function)
if not future.cancelled(): # 取消任务前检查是否已被取消
future.cancel()
```
在Python标准库中,`cancel()`方法对于非守护线程效果有限,因为守护线程默认会被系统清理掉,而不必手动取消。
python 多线程执行2个while
在Python中,可以使用threading模块来实现多线程的操作。要实现同时执行两个while循环,可以创建两个线程分别执行两个while循环的任务。
首先,我们需要导入threading模块,并定义两个函数,用来表示两个while循环的任务。例如:
```python
import threading
def task1():
while True:
# 执行任务1的操作
def task2():
while True:
# 执行任务2的操作
```
然后,我们可以创建两个线程分别执行这两个任务。例如:
```python
thread1 = threading.Thread(target=task1)
thread2 = threading.Thread(target=task2)
thread1.start() # 启动线程1
thread2.start() # 启动线程2
```
这样,两个线程就会同时执行各自的任务,实现了同时执行两个while循环。
需要注意的是,如果两个while循环的任务都是无限循环,那么这两个线程就会一直执行下去,除非程序被手动停止。如果希望在某个条件达到时终止循环,可以在while循环内部添加相应的判断语句来控制循环的结束。
同时,由于多线程存在共享资源的问题,如果两个任务涉及到共享的数据,需要考虑线程安全的问题,例如使用锁机制来避免多个线程同时对共享数据进行修改。
阅读全文
相关推荐
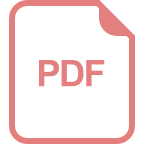
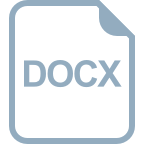
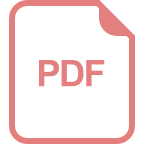













