Android 实现天气预报
时间: 2024-03-29 15:33:25 浏览: 122
Android实现天气预报可以通过以下步骤进行:
1. 获取天气数据:首先需要从天气数据源获取实时的天气数据。可以使用第三方的天气API,如和风天气API、心知天气API等,通过发送HTTP请求获取天气数据的JSON格式。
2. 解析天气数据:获取到的天气数据是JSON格式的,需要对其进行解析,提取出需要的天气信息,如温度、湿度、风力等。可以使用Android提供的JSON解析库,如Gson或者JsonReader来解析JSON数据。
3. 设计界面:根据需求设计天气预报界面,可以使用Android提供的布局组件来构建界面,如LinearLayout、RelativeLayout等。可以根据天气数据的不同信息,在界面上展示相应的天气情况。
4. 显示天气信息:将解析得到的天气信息显示在界面上,可以使用TextView或者ImageView等组件来展示温度、湿度、风力等信息。可以根据不同的天气情况,使用不同的图标或者背景来表示。
5. 更新天气数据:为了保持实时性,可以设置一个定时器或者使用后台服务来定期更新天气数据。可以根据需求设置更新频率,如每小时更新一次。
相关问题
Android实现天气预报界面
要实现天气预报界面,你需要完成以下几个步骤:
1. 获取天气数据:你可以使用第三方的天气API来获取天气数据,比如和风天气、心知天气等等。这些API提供了基于城市、经纬度等方式获取天气数据的接口,你可以选择适合自己的方式进行调用。
2. 设计界面:你可以使用Android Studio提供的布局控件进行设计,比如LinearLayout、RelativeLayout、ConstraintLayout等。你可以根据自己的需求选择不同的布局方式。
3. 显示天气数据:你需要将获取到的天气数据显示在UI上。你可以使用TextView、ImageView等控件进行显示。
4. 添加动态效果:如果你想让你的天气预报界面更加有趣,你可以添加一些动态效果,比如渐变背景、动态图标等。你可以使用Android提供的动画和过渡效果进行实现。
总之,实现天气预报界面需要你具备一定的Android开发经验和UI设计能力,同时需要你对天气数据有一定的了解。如果你是初学者,可以先从简单的UI布局开始学习,然后逐步增加功能和效果。
利用android实现天气预报代码
以下是一个简单的 Android 天气预报应用的代码示例:
1. 创建一个新的 Android 项目,并在 MainActivity.java 文件中添加以下代码:
```java
public class MainActivity extends AppCompatActivity {
private TextView mCityTextView;
private TextView mTemperatureTextView;
private TextView mDescriptionTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mCityTextView = findViewById(R.id.city_textview);
mTemperatureTextView = findViewById(R.id.temperature_textview);
mDescriptionTextView = findViewById(R.id.description_textview);
getWeatherData();
}
private void getWeatherData() {
String url = "https://api.openweathermap.org/data/2.5/weather?q=London,uk&appid=YOUR_API_KEY&units=metric";
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest(Request.Method.GET, url, null,
new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
try {
JSONObject main = response.getJSONObject("main");
JSONArray weather = response.getJSONArray("weather");
JSONObject weatherObject = weather.getJSONObject(0);
String city = response.getString("name");
String temperature = main.getString("temp");
String description = weatherObject.getString("description");
mCityTextView.setText(city);
mTemperatureTextView.setText(temperature + "°C");
mDescriptionTextView.setText(description);
} catch (JSONException e) {
e.printStackTrace();
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Toast.makeText(MainActivity.this, "Error", Toast.LENGTH_SHORT).show();
}
});
RequestQueue requestQueue = Volley.newRequestQueue(this);
requestQueue.add(jsonObjectRequest);
}
}
```
2. 在 activity_main.xml 文件中添加以下代码:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/city_textview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="30sp"
android:textStyle="bold"
android:layout_centerHorizontal="true"
android:layout_marginTop="50dp"/>
<TextView
android:id="@+id/temperature_textview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="70sp"
android:textStyle="bold"
android:layout_below="@+id/city_textview"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"/>
<TextView
android:id="@+id/description_textview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20sp"
android:textStyle="italic"
android:layout_below="@+id/temperature_textview"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"/>
</RelativeLayout>
```
3. 在 AndroidManifest.xml 文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
4. 在 build.gradle 文件中添加以下依赖项:
```gradle
dependencies {
implementation 'com.android.volley:volley:1.1.1'
}
```
5. 将 YOUR_API_KEY 替换为您的 OpenWeatherMap API 密钥。
这个示例使用了 Volley 库来处理网络请求并从 OpenWeatherMap API 中获取天气数据。它显示了城市名、温度和天气描述。您可以根据需要对代码进行修改和扩展。
阅读全文
相关推荐
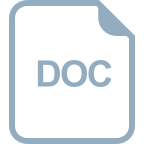
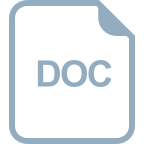
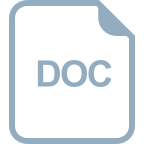
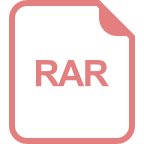
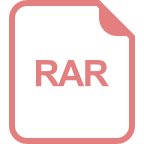
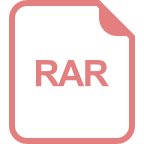



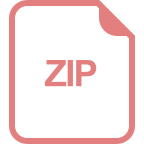
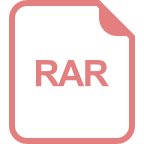
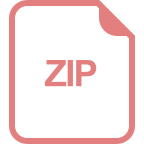



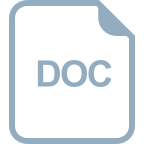