nlohmann::json 转换为std::string
时间: 2024-09-30 10:14:50 浏览: 41
nlohmann::json是一个流行的C++ JSON库,它提供了一个方便的方式来处理JSON数据。如果你有一个`nlohmann::json`类型的对象,想要将其转换为`std::string`(即字符串),你可以简单地调用该对象的`dump()`或`to_string()`方法。这里是一个例子:
```cpp
#include <nlohmann/json.hpp>
#include <string>
// 假设你已经有一个名为json_obj的nlohmann::json对象
nlohmann::json json_obj;
// 使用dump()方法将JSON转换为字符串(包含缩进和新行)
std::string jsonString = json_obj.dump();
// 或者使用to_string()方法简化输出(不带额外空格和换行)
std::string jsonStringWithoutIndent = json_obj.to_string();
```
`dump()`方法会返回一个格式化的字符串,而`to_string()`方法则返回一个紧凑的字符串形式。
相关问题
将std::string转换为nlohmann::json
在C++中,`std::string`通常代表字符串类型,而`nlohmann::json`是一个流行的JSON库,用于处理JavaScript Object Notation(JSON)数据结构。要将`std::string`转换成`nlohmann::json`对象,你需要使用`nlohmann::json`库提供的解析功能。
以下是如何完成这个转换的一个例子:
```cpp
#include <nlohmann/json.hpp> // 引入nlohmann::json头文件
// 假设你有一个包含JSON字符串的std::string
std::string jsonString = "{\"name\":\"John\", \"age\":30}";
// 使用nlohmann::json的parse方法将字符串转换为json对象
nlohmann::json jsonObject;
try {
jsonObject = nlohmann::json::parse(jsonString);
} catch (const std::exception& e) {
// 处理解析错误
std::cerr << "Error parsing JSON: " << e.what() << '\n';
}
// 现在你可以像操作普通的json对象一样操作(jsonObject)
```
在这个例子中,如果`jsonString`有效并且是有效的JSON格式,那么`jsonObject`就会被成功地初始化。如果JSON字符串格式不正确,`parse`方法会抛出异常。
``` nlohmann::json ```const char 转 nlohmann::json
`nlohmann::json` 是一个轻量级的 JSON 库,由 Joachim Buhler 创作,专为 C++ 编程设计,它提供了一种方便的方式来处理 JSON 数据。这个库是非依赖性的,即不需要外部链接,非常适合在嵌入式系统或资源受限环境中使用。
如果你想要将 `const char*` 类型的字符串转换为 `nlohmann::json` 对象,你可以使用 `nlohmann::json::parse()` 函数。这是一个基本的例子:
```cpp
#include <nlohmann/json.hpp>
// 假设你有一个 const char* 字符串,存储了 JSON 格式的数据
const char* jsonString = "{\"name\":\"John\", \"age\":30}";
// 使用 parse() 函数解析字符串
nlohmann::json jsonObject;
try {
jsonObject = nlohmann::json::parse(jsonString);
} catch (const std::exception& e) {
// 处理解析错误
std::cout << "Error parsing JSON: " << e.what() << std::endl;
}
// 现在 jsonObject 就是一个有效的 nlohmann::json 对象
```
在这个例子中,如果输入的字符串是有效的 JSON,`parse()` 会返回一个 `json` 对象;如果格式不正确,则抛出异常。
阅读全文
相关推荐
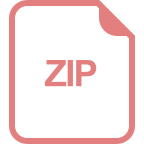
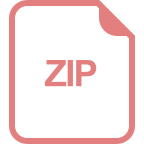
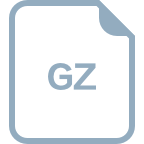














