python实现pdf转图片带界面
时间: 2023-05-29 15:06:58 浏览: 173
作为一个AI语言模型,我只能提供一些思路和建议,具体的代码实现还需要开发者自行编写。
思路:
1. 使用Python中的pdf2image库将pdf文件转换为图片;
2. 使用Python中的Tkinter库创建界面;
3. 使用Python中的Pillow库处理图片,并将其显示在界面上。
建议:
1. 在安装pdf2image库之前,需要先安装poppler软件,因为pdf2image库需要使用poppler进行pdf转换。可以在命令行中使用以下命令安装:
```
sudo apt-get install poppler-utils
```
2. 使用Tkinter库创建界面时,可以参考以下代码:
```python
from tkinter import *
from tkinter import filedialog
from PIL import Image, ImageTk
import os
import pdf2image
class PDF2ImageGUI:
def __init__(self, root):
self.root = root
self.root.title("PDF to Image Converter")
# 创建文件选择按钮
self.select_button = Button(self.root, text="Select PDF", command=self.select_pdf)
self.select_button.pack()
# 创建转换按钮
self.convert_button = Button(self.root, text="Convert", command=self.convert_pdf)
self.convert_button.pack()
# 创建图片显示区域
self.image_frame = Frame(self.root)
self.image_frame.pack()
def select_pdf(self):
# 打开文件选择对话框
self.pdf_file = filedialog.askopenfilename(filetypes=[("PDF Files", "*.pdf")])
def convert_pdf(self):
# 将pdf文件转换为图片
images = pdf2image.convert_from_path(self.pdf_file)
# 在界面上显示图片
for i, image in enumerate(images):
# 将PIL Image对象转换为Tkinter PhotoImage对象
img = ImageTk.PhotoImage(image)
# 创建Label并显示图片
label = Label(self.image_frame, image=img)
label.image = img
label.pack(side=LEFT)
# 将图片保存到本地
image.save(f"{os.path.splitext(self.pdf_file)[0]}_{i+1}.png")
if __name__ == "__main__":
root = Tk()
app = PDF2ImageGUI(root)
root.mainloop()
```
这段代码创建了一个有文件选择按钮和转换按钮的界面,当用户选择PDF文件后,点击转换按钮会将PDF文件转换为图片,并在界面上显示图片。同时,也会将图片保存到本地。需要注意的是,这里使用了pdf2image库进行转换,并且在保存图片时使用了os库获取文件名和路径。
3. 在使用Pillow库处理图片时,需要注意以下几点:
- PIL Image对象不能直接显示在Tkinter界面上,需要将其转换为Tkinter PhotoImage对象;
- Tkinter PhotoImage对象在创建后需要保留对其的引用,否则会被垃圾回收机制回收;
- 在保存图片时,需要使用Image对象的save方法,并指定保存的文件名和路径。
希望这些思路和建议能帮助到你实现PDF转图片的界面程序。
阅读全文
相关推荐
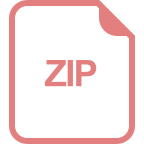
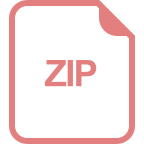
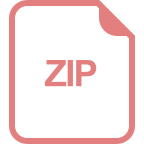















